If you’re customizing your site and performing specific actions for certain content, it may be useful to test if your current WordPress page is a custom post type.
This way, you can display customized content, change the layout, or take any other conditional action you may need.
This post will help you identify whether your current page is a custom post type. It contains some basic PHP code that you can insert into your functions.php
file to accomplish this goal.
Key Takeaways
- You can determine whether your post is a custom post type by adding custom code to the functions.php file.
- This custom code can contain logic to display the current post type, letting you confirm whether it is a custom post type or not.
- Another approach is to compare the current post’s type to every default WordPress post type to get a more immediate answer.
Why Test if the Current WordPress Page Is a Custom Post Type?
You’ll likely want to test whether your current page is a custom post type to perform actions based on this condition.
For example, you may want to slightly modify the footer on certain custom post types or display a text box at the top of certain other custom post types.
Learning to identify custom post types programmatically allows you to perform these actions on the correct post types.
How to Test if the Current Page is a Custom Post Type in WordPress?
There are multiple ways to determine if any given post is a custom post type. These are 2 methods you may consider using for your site.
Method #1: Use the get_post_type Function to Display the Type of the Current Post
The get_post_type function retrieves a text string with the post type of the current post or any specific post you want. It queries the WordPress database to determine the type of a given post.
get_post_type()
can be very useful in multiple scenarios, such as applying specific layouts or styles based on the post type or displaying different sidebars or custom fields for different post types.
In this case, we’ll use it to determine the type of the current post. If you know the type of the current post, you can determine whether it is a custom post type or not.
Place the following PHP snippet in your theme’s functions.php
file to check the type of the current post and display its type in a textbox:
/**
* Determines the type of post and displays it at the beginning of a single post
*
* @return string
*/
if ( ! function_exists( 'detect_and_display_post_type' ) ) :
function detect_and_display_post_type ($content) {
$current_post_type = get_post_type();
if ( is_single() ) {
$content_type_text =
'<div
style="background-color: #333333;
color: #ffffff;
font-size: 22px;
padding: 20px;
margin-top: 30px;
margin-bottom: 30px;
border-radius: 8px;
line-height: 1.6;
text-align: center;"
class="welcome-box">
This post belongs to the following post type: ' . $current_post_type .
'</div>';
$content = $content_type_text . $content;
}
return $content;
}
endif;
add_filter('the_content', 'detect_and_display_post_type');
After placing this snippet in functions.php
and saving the changes, you should see this textbox appear at the top of the content of every single post on your site:
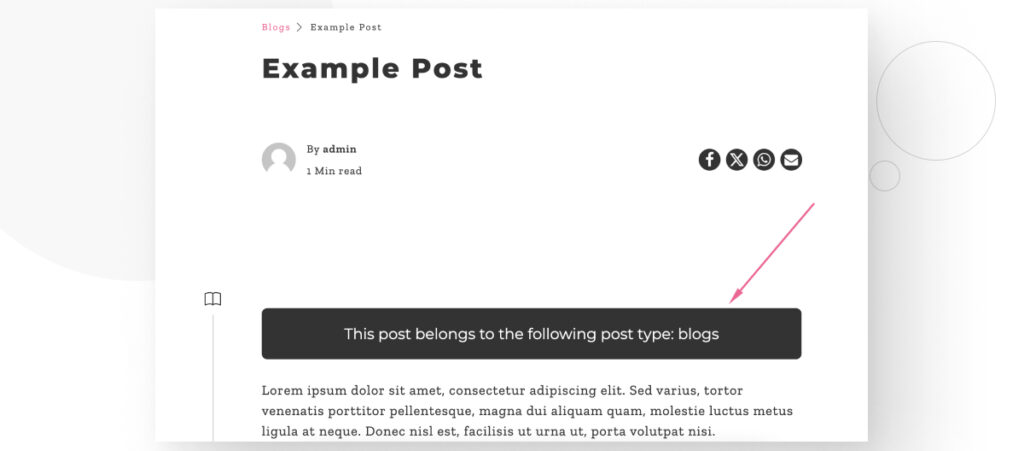
In this case, this post belongs to one of our custom post types, blogs.
Method #2: Use a Function to Compare the Current Post’s Type to All the Default Post Types
A more direct approach is to compare the current post type to every built-in post type that all WordPress installations come with.
To do that, you can create a custom function that captures the current post type in a variable. This variable will be a string with the result of get_post_type()
.
You can compare this string to strings corresponding to every default post type. If your string corresponds to the strings of any default post type, then the post is not a custom post type. If your string doesn’t correspond, then it is a custom post type.
Place the following PHP snippet in your theme’s functions.php
:
/**
* Check if the current post is a custom post type.
*
* @return string
*/
if ( ! function_exists( 'is_custom_post_type' ) ) :
function is_custom_post_type($content) {
// Get the current post type.
$post_type = get_post_type();
// Check if the post type exists and is not one of the default WordPress post types.
if ($post_type && !in_array($post_type, ['post', 'page', 'attachment', 'revision', 'nav_menu_item'], true)) {
$post_type_text =
'<div
style="background-color: #333333;
color: #ffffff;
font-size: 22px;
padding: 20px;
margin-top: 30px;
margin-bottom: 30px;
border-radius: 8px;
line-height: 1.6;
text-align: center;"
class="welcome-box">
This is not a custom post type.
</div>';
$content = $post_type_text . $content;
return $content;
} else {
$post_type_text =
'<div
style="background-color: #333333;
color: #ffffff;
font-size: 22px;
padding: 20px;
margin-top: 30px;
margin-bottom: 30px;
border-radius: 8px;
line-height: 1.6;
text-align: center;"
class="welcome-box">
This is a custom post type.
</div>';
$content = $post_type_text . $content;
return $content;
}
}
endif;
add_filter('the_content', 'is_custom_post_type');
If your post is a custom post, you will see this at the beginning of your posts:
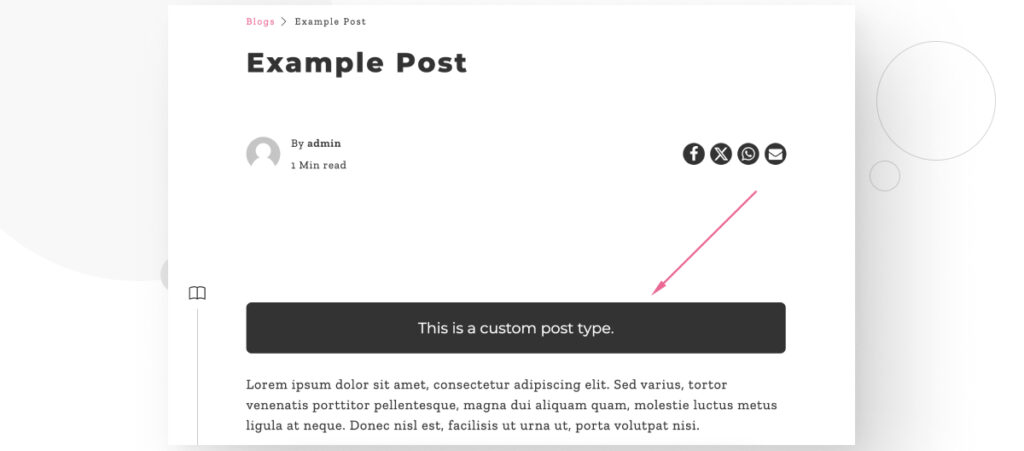
Otherwise, you will see this message confirming that it is not a custom post type:
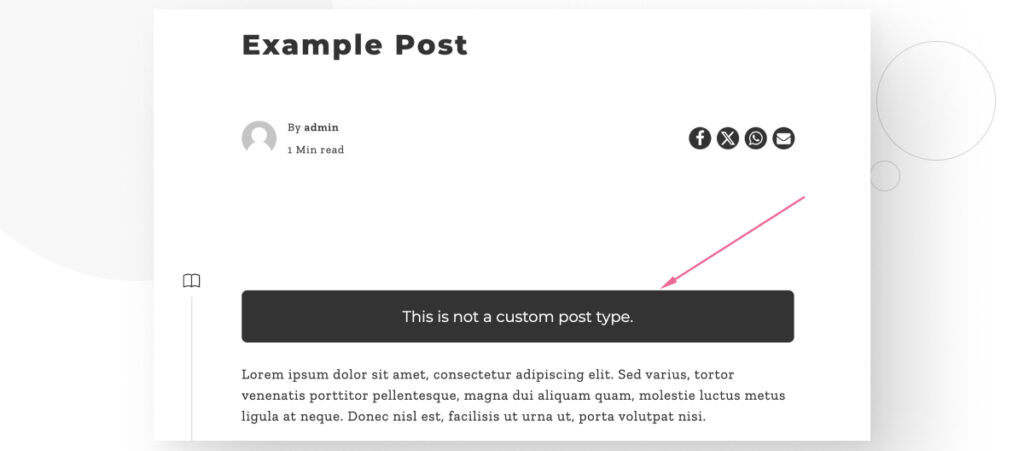
While you may not necessarily want to display a textbox, this function gives you a starting point to perform actions based on whether a post belongs to a custom post type.
Test if Your Current WordPress Page Is a Custom Post Type
As you can see, you can determine whether a WordPress page is a custom post type by adding a custom relatively straightforward function to your functions.php
file.
One option is to display the current post type on the page. This will allow you to confirm whether it is a custom post type.
Another, more direct method is to include logic into your function to compare the current post type to every default post type. This will allow you to receive a more immediate answer as to whether it is a custom post type or not.
If you found this post useful, read our blog and developer resources for more insights and guides!