Migrar contenido en WordPress puede ser abrumador, especialmente cuando se lida con una combinación de bloques personalizados y plugins de terceros. Sin embargo, utilizando WP All Import y la función parse_blocks()
de WordPress, es posible transformar eficientemente bloques personalizados en bloques nativos, creando una experiencia de contenido más fluida y manejable.
Si no se importan adecuadamente, estos bloques pueden interrumpir el estilo de un tema, provocando una estética inconsistente y una estructura de contenido desordenada. Por lo tanto, es muy importante realizar correctamente la importación.
Exploremos cómo hacerlo.
El Desafío de los Bloques Personalizados
Una de las principales razones para transformar bloques personalizados a bloques nativos es el desafío que representan para el mantenimiento a largo plazo. Además, los bloques personalizados introducidos por plugins de terceros a menudo rompen el estilo y la consistencia estética del sitio.
En un proyecto reciente, el cliente tenía varios bloques personalizados que no se alineaban con las plantillas y patrones que creamos (WordPress FSE). Nuestras plantillas proporcionaban un aspecto estructurado, coherente para el sitio, pero los bloques personalizados no eran compatibles con eso.
Mantener estos bloques de terceros habría socavado nuestro esfuerzo por lograr un diseño web bien organizado y consistentemente visual.
Convirtiendo estos bloques a bloques nativos, obtuvimos mejor control sobre la apariencia y funcionalidad del contenido. Esto aseguró que se ajustara a la guía de estilo y mejoró la consistencia general del sitio.
¿Por qué WP All Import?
Hemos utilizado WP All Import con gran éxito en múltiples proyectos ahora.
Sus robustas características y capacidad para manejar importaciones complejas lo han convertido en nuestra primera opción al importar grandes volúmenes de contenido. Una de sus principales fortalezas es su flexibilidad, permitiéndonos escribir scripts personalizados para manipular datos durante el proceso de importación.
Esta personalización resulta útil al transformar bloques personalizados en bloques nativos. Para esta migración en particular, las capacidades de scripts de WP All Import nos permitieron ajustar finamente cómo se manejaba cada bloque durante la importación.
Aprovechando parse_blocks() para la Transformación de Bloques
Inicialmente, la estrategia para identificar y reemplazar bloques se basó en expresiones regulares (regex).
Este enfoque funcionó para bloques más sencillos, como convertir un bloque espaciador de Genesis en un bloque espaciador nativo de WordPress.
Sin embargo, a medida que los bloques se volvieron más complejos, el uso de regex resultó insuficiente. Al enfrentarme con bloques más robustos, comencé a buscar alternativas y me encontré con la función parse_blocks()
en la documentación de WordPress.
parse_blocks()
analiza el contenido de las publicaciones en un array de objetos de bloques, proporcionando acceso a información crucial como nombres de bloques, atributos, HTML interno y bloques anidados (innerBlocks).
Esto me permitió desarrollar condiciones y lógica basadas en la estructura del bloque, lo que hizo mucho más fácil transformar bloques personalizados complejos en bloques nativos.
Convertir Bloques Genesis a Nativos Usando PHP: 2 Ejemplos
Ejemplo #1: El Resultado Final
El proceso de transformación, aunque efectivo, ha sido complicado debido a la estructura única de cada bloque.
No hay dos bloques personalizados exactamente iguales, lo que significa que cada uno requiere una depuración exhaustiva para entender su estructura y determinar cómo reemplazarlo mejor. El proceso de averiguar todo esto tomó más de 16 horas de trabajo.
Aquí tienes un ejemplo de cómo lucen los bloques después de ser transformados de Genesis a bloques nativos.
Antes (Bloque Personalizado)
<!-- wp:columns {"columns":2} -->
<div class="wp-block-genesis-blocks-gb-columns gb-layout-columns-2 gb-2-col-equal alignwide"><div class="gb-layout-column-wrap gb-block-layout-column-gap-2 gb-is-responsive-column"><!-- wp:column -->
<div class="wp-block-genesis-blocks-gb-column gb-block-layout-column"><div class="gb-block-layout-column-inner"><!-- wp:heading {"level":5} -->
<h5>Lorem Ipsum</h5>
<!-- /wp:heading -->
<!-- /wp:genesis-blocks/gb-column -->
<!-- wp:column -->
<div class="wp-block-genesis-blocks-gb-column gb-block-layout-column"><div class="gb-block-layout-column-inner"><!-- wp:heading {"level":5} -->
<h5>Lorem Ipsum</h5>
<!-- /wp:heading -->
<!-- /wp:genesis-blocks/gb-column --></div></div>
<!-- /wp:columns -->
Después (Bloque Nativo)
<!-- wp:columns -->
<div class="wp-block-columns"><!-- wp:column -->
<div class="wp-block-column"><!-- wp:heading {"level":5} -->
<h5 class="wp-block-heading">Lorem Ipsum</h5></div>
<!-- /wp:heading -->
<!-- wp:column -->
<div class="wp-block-column"><!-- wp:heading {"level":5} -->
<h5 class="wp-block-heading">Lorem Ipsum</h5>
<!-- /wp:heading --></div>
<!-- /wp:column --></div>
<!-- /wp:columns -->
Este ejemplo muestra el resultado final de transformar un diseño de bloque de columna Genesis en un bloque de columnas nativo de WordPress, asegurando que todo HTML, atributos y bloques internos se preserven y migren correctamente.
Ejemplo #2: El Proceso Paso a Paso
Exploremos un segundo ejemplo más detallado, mostrando el proceso paso a paso de cómo lograr esta transformación usando WP All Import y parse_blocks()
. Aquí está el código para convertir Columnas Genesis a Columnas Nativas:
/**
* Convertir bloques de Genesis a bloques nativos de WordPress.
*
* Esta función analiza el contenido, identifica bloques de Genesis (Columns y Column)
* y los convierte en bloques nativos de WordPress.
*
* @param string $content El contenido original que contiene bloques de Genesis.
* @return string El contenido con los bloques de Genesis convertidos a bloques nativos.
*/
function convert_genesis_blocks_to_core( $content ) {
// Analizar el contenido en bloques.
$blocks = parse_blocks( $content );
// Convertir los bloques recursivamente.
$converted_blocks = convert_blocks_recursively( $blocks );
// Serializar los bloques de regreso al contenido y devolver.
return serialize_blocks( $converted_blocks );
}
/**
* Convertir recursivamente bloques personalizados a bloques nativos.
*
* Esta función recorre los bloques analizados y aplica transformaciones a los bloques de Genesis.
*
* @param array $blocks Un array de objetos de bloques analizados.
* @return array El array transformado de objetos de bloques.
*/
function convert_blocks_recursively( $blocks ) {
$converted_blocks = array();
foreach ( $blocks as $block ) {
// Convertir bloques de columna Genesis a columnas nativas
if ( 'genesis-blocks/gb-columns' === $block['blockName'] ) {
$converted_blocks[] = convert_genesis_columns_block( $block );
} elseif ( 'genesis-blocks/gb-column' === $block['blockName'] ) {
$converted_blocks[] = convert_genesis_column_block( $block );
} else {
// Convertir recursivamente bloques internos
if ( ! empty( $block['innerBlocks'] ) ) {
$block['innerBlocks'] = convert_blocks_recursively( $block['innerBlocks'] );
}
$converted_blocks[] = $block;
}
}
return $converted_blocks;
}
/**
* Convertir bloque de Columnas de Genesis a bloque de Columnas nativas.
*
* Esta función mapea atributos y estructura del bloque de Columnas de Genesis a atributos de Columnas nativas.
*
* @param array $block Los datos del bloque de Columnas de Genesis.
* @return array El bloque transformado de Columnas nativas.
*/
function convert_genesis_columns_block( $block ) {
$attrs = array( 'columns' => $block['attrs']['columns'] ?? 2 );
// Convertir recursivamente bloques internos (Columnas de Genesis)
$inner_blocks = convert_blocks_recursively( $block['innerBlocks'] );
return array(
'blockName' => 'core/columns',
'attrs' => $attrs,
'innerBlocks' => $inner_blocks,
'innerHTML' => '', // Se regenerará automáticamente
'innerContent' => array(),
);
}
/**
* Convertir bloque de Columna de Genesis a bloque de Columna nativa.
*
* Esta función convierte Columnas individuales de Genesis en Columnas nativas de WordPress.
*
* @param array $block Los datos del bloque de Columna de Genesis.
* @return array El bloque transformado de Columna nativa.
*/
function convert_genesis_column_block( $block ) {
// Convertir recursivamente bloques internos dentro de la Columna
$inner_blocks = convert_blocks_recursively( $block['innerBlocks'] );
return array(
'blockName' => 'core/column',
'attrs' => array(), // Sin atributos especiales para el bloque de Columna nativa
'innerBlocks' => $inner_blocks,
'innerHTML' => '', // Se regenerará automáticamente
'innerContent' => array(),
);
}
Este ejemplo muestra cómo transformar un diseño de bloque de columna Genesis en un bloque de columnas nativo de WordPress asegurando que todo HTML, atributos, y bloques internos se preserven y migren correctamente.
Integrando el Código con WP All Import
Una vez que hayas creado tu código de transformación de bloques, es hora de ponerlo en funcionamiento dentro de WP All Import. El proceso es simple y se puede realizar durante la configuración de la importación.
En el tercer paso de crear una nueva importación (la interfaz de “Arrastrar y Soltar”), normalmente mapearías tus campos de contenido. En lugar de usar el campo estándar {content[1]}
para tu contenido de publicación, podés llamar la función personalizada directamente para manejar la transformación de bloques.
Por ejemplo, usa:
[convert_genesis_blocks_to_core({content[1]})]
Esto aplicará tu lógica de transformación al contenido antes de que se importe, asegurando que tus bloques personalizados sean convertidos a bloques nativos durante el proceso de importación.
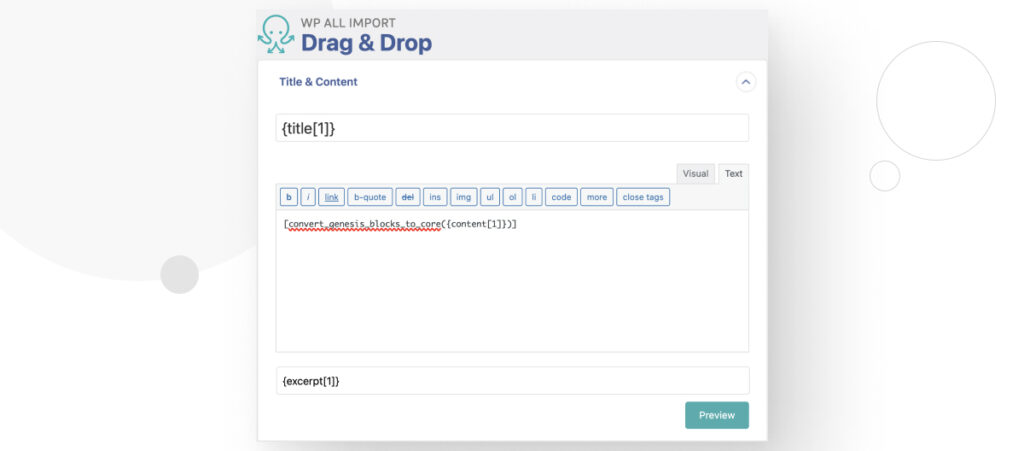
¿Dónde Definir la Función para Convertir Bloques Personalizados a Bloques Nativos?
WP All Import te permite definir funciones personalizadas directamente dentro de la interfaz.
Desplazate hacia abajo en el mismo paso de “Arrastrar y Soltar” hasta que encuentres la sección “Editor de Funciones”. Aquí, podés pegar el código PHP para la función convert_genesis_blocks_to_core()
. Una vez agregado, WP All Import lo reconocerá y lo aplicará al contenido según lo configurado.
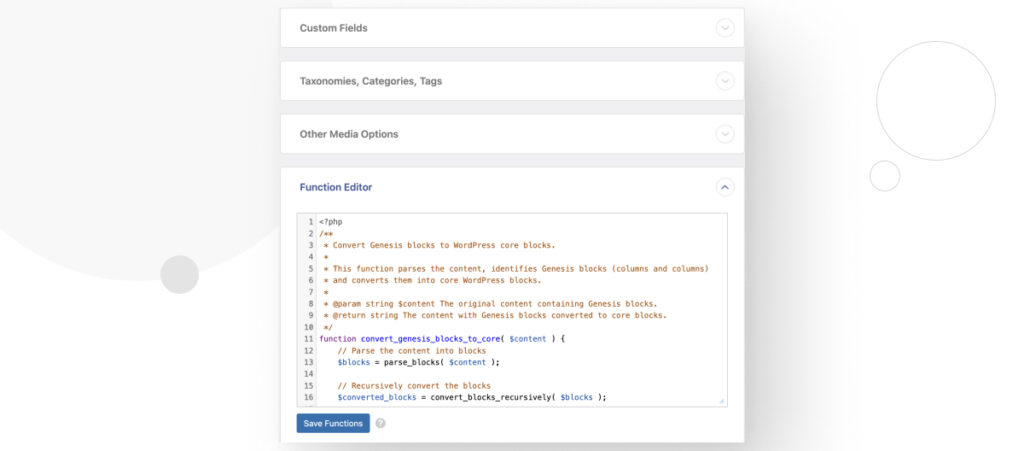
Para obtener más orientación detallada, podés consultar la documentación oficial de WP All Import sobre el uso de PHP en línea.
Beneficios de Usar Bloques Nativos
Los beneficios de esta transformación van más allá de la apariencia.
Al usar bloques nativos de WordPress junto con nuestros bloques personalizados, hemos minimizado nuestra dependencia de plugins de terceros. Esto mejora el rendimiento ya que se cargan menos scripts externos en el frontend y además nos da control total sobre el estilo y comportamiento de los bloques.
Personalizar un bloque nativo es mucho más fácil que adaptar un bloque personalizado de otro.
Además, la experiencia del cliente permanece fluida, ya que los bloques nativos son intuitivos y bien conocidos dentro del ecosistema WordPress.
Pruebas y Asegurar la Integridad de los Datos
Como con cualquier migración grande, asegurar la integridad del contenido es importante. Realizamos múltiples migraciones locales, procesando más de 1,700 publicaciones en total. Para asegurar la precisión, revisamos aproximadamente una publicación de cada treinta, verificando manualmente que los datos y el formato se preservaran correctamente después de la transformación.
Estas pruebas nos ayudaron a mantener la confianza de que ningún contenido se perdió o dañó durante el proceso.
Transformar Bloques Personalizados en Bloques Nativos Optimiza la Gestión de Contenidos
Al transformar bloques personalizados en bloques nativos, hemos hecho el sitio del cliente más eficiente, consistente y fácil de mantener.
WP All Import y parse_blocks()
resultaron herramientas invaluables en este proceso, permitiendo una solución flexible y escalable para manejar migraciones de contenido complejas.
Para desarrolladores enfrentando desafíos similares, este método no solo asegura contenido a futuro sino que también reduce significativamente la carga de gestionar plugins de terceros.
Si encontraste útil este post, leé nuestro blog y recursos de desarrolladores para más insights y guías!
Artículos relacionados
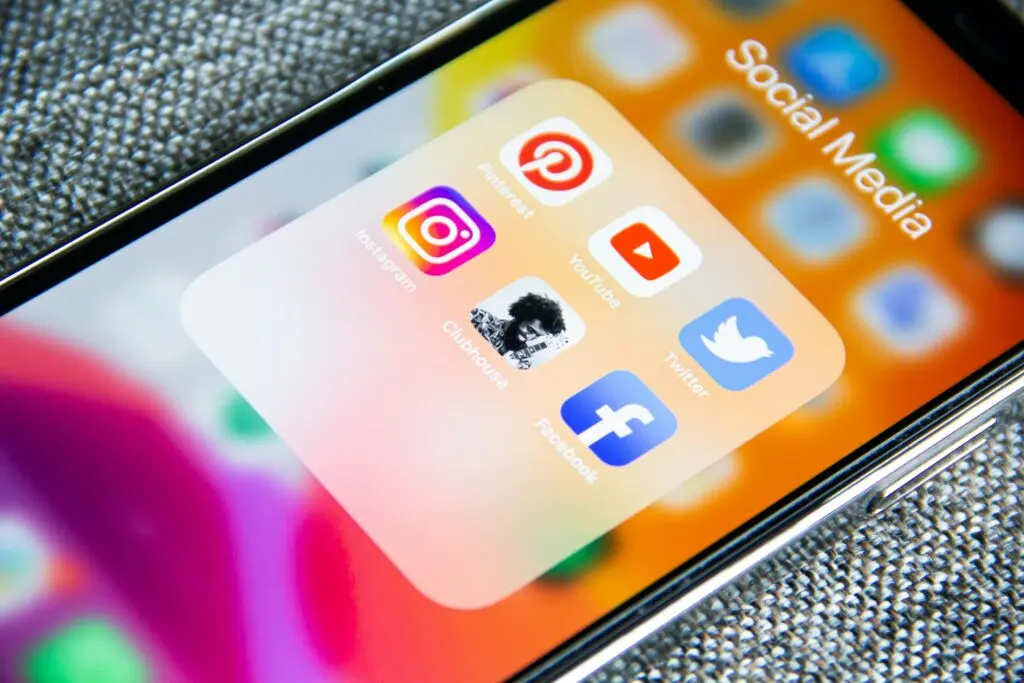
Plugins / 4 min de lectura
Plugins / 4 min de lectura
Social Share for Devs: Revision del Plugin de WordPress
Social Share for Devs es un plugin gratuito y sencillo para botones de compartir en redes sociales. Los plugins de WordPress para compartir en redes sociales simplifican el proceso de…
Read More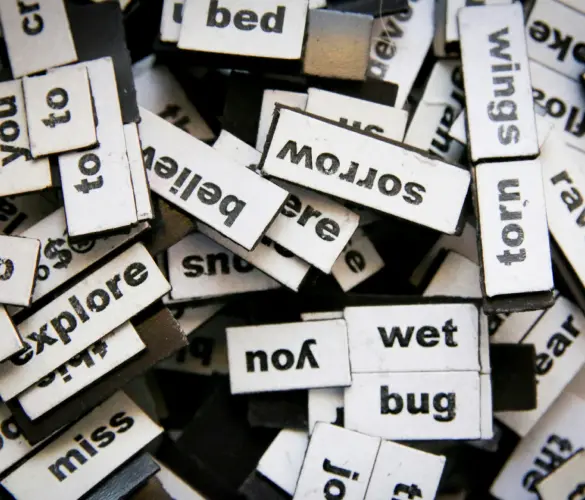
Plugins / 5 min de lectura
Plugins / 5 min de lectura
Cómo usar el plugin Lorem Ipsum Scanner para WordPress
Diseñadores y desarrolladores usan el texto “lorem ipsum” como marcador de posición para visualizar cómo se verá el contenido final en el sitio web terminado. Sin embargo, a pesar de…
Read More