- Blogs
- WordPress 101
- WordPress is_page Function: What it Is and How to use it
WordPress 101 / 5 min read
WordPress is_page Function: What it Is and How to use it
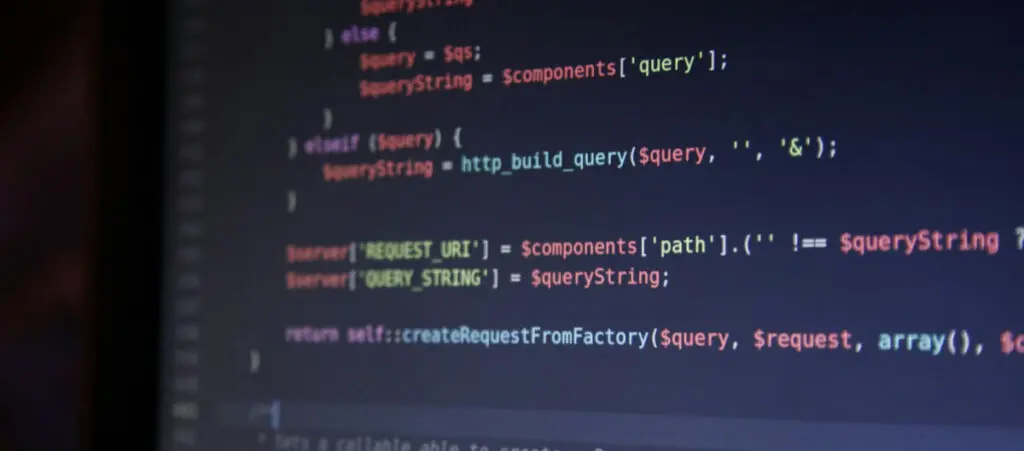
Sometimes, when developing custom functions, themes, or plugins, it’s useful to determine whether a Page is being displayed. That’s when the WordPress function is_page comes into play.
is_page
allows developers to determine whether the page being displayed is of the post type Page, letting you run code conditionally based on parameters like the post ID, page title, and page slug.
Let’s explore what the function is_page
does and how to use it in WordPress.
Key Takeaways
- is_page is a WordPress conditional tag that determines whether the user is on a single page.
- Is_page takes in either no parameters or an integer (post ID), string (page slug or page title), an array of post IDs, or an array of page slugs/titles.
- With is_page, you can run code conditionally on all pages or specific pages, making it very useful for theme and plugin development.
What is the is_page WordPress Function?
The is_page
function in WordPress is a conditional tag that checks whether the current page is a single Page.
Pages are a WordPress post type that shows “static” content: content that doesn’t change frequently. Examples include informational web pages such as “About Us” and “‘Privacy Policy.” Pages are not listed chronologically and don’t use tags or categories like posts often do.
Depending on whether the current page is a single Page and the provided arguments, is_page
returns a Boolean value: true
or false
.
Developers commonly use the is_page
function in WordPress themes and plugins to conditionally display different content or styles based on the current page being viewed. For example, you might use it to add a specific CSS class to the body tag of a particular Page or to display a custom sidebar widget only on certain pages.
It’s important to note that is_page
only checks for single Pages, not hierarchical pages (child pages). To check for child pages, you would use the is_page_template
conditional tag along with the appropriate page template filename.
How Does is_page Work?
Here’s the definition of is_page
:
is_page( int|string|int[]|string[] $page = ” ): bool
The function works with or without parameters and returns a Boolean value.
Without any arguments, is_page
will simply return true if the current page being viewed is any Page (as opposed to a post, archive, or other type of page).
You may also use is_page
with arguments such as a page ID, an array of page IDs, the page’s slug or title, an array of page slugs and titles, or a WP_Post
object.
Page ID as a Parameter
You can pass the ID of a specific page as an integer argument. For example, is_page(12)
will return true if the current page being viewed has the ID of 12, and false
otherwise.
Passing an array of page IDs works, too. Consider the following array:
is_page( array( 12, 25, 102 ) )
is_array
will return true if the current page matches any of the provided page IDs.
Page Slug or Page Title as a Parameter
You can pass the slug (the permalink-friendly part of the URL) or post_title
of a page as a string argument.
For example, is_page('about')
will return true if the current page being viewed has the slug about.
is_page('Our Careers')
will return true if the current page being viewed has Our Careers as its post_title.
Alternatively, you may pass an array of slugs or page titles, like:
is_page( array( 'about-us', 'Our Careers' ) )
This condition will return true if the current page’s slug matches any of the provided slugs or titles.
Finally, you can mix strings and integers in the same array. The function will match the elements of the array to the appropriate field (post ID, post title, or slug).
Page Object
It’s also possible to pass a WP_Post
object or an array of page IDs as an argument to is_page
.
How to Use the is_page WordPress Function
Let’s do a simple test for the is_page
function. For this test, we’ll display a textbox on every page of our WordPress site.
To do that, we’ll add the following code to our theme’s functions.php
file:
if ( ! function_exists ( 'display_text_box' ) ) :
function display_text_box() {
if ( is_page() ) : // Checks if the page is a single page
echo '<p style="position: absolute;
font-size: 25px;
top: 180px;
right: 100px;
padding: 10px;
background-color: #000000;
color: #FFFFFF;">This is a page. Don\'t panic!</p>';
endif;
}
endif;
add_action( 'wp_footer', 'display_text_box' );
After adding this snippet, every Page in your site should display a text box with the text This is a page. Don’t panic!:
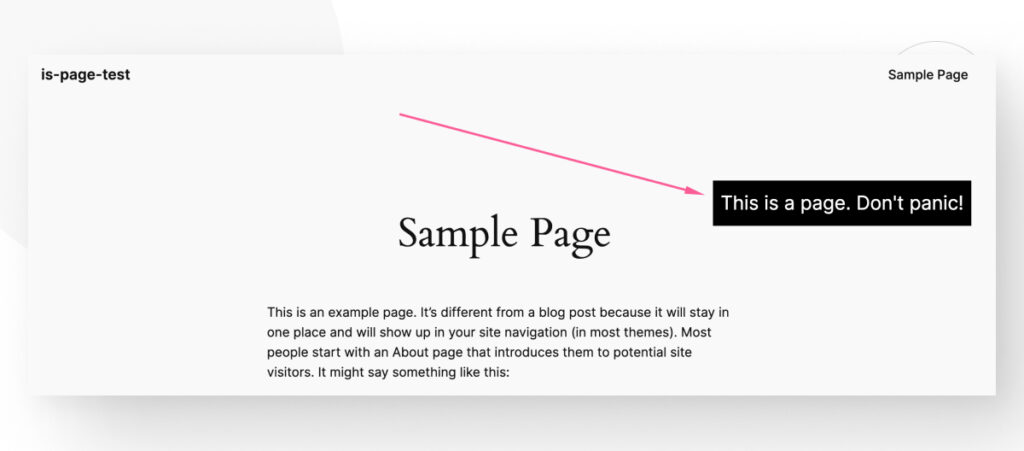
You can also modify the code to check for a more specific condition, such as a page with the about-us
slug:
if ( ! function_exists ( 'display_text_box_about' ) ) :
function display_text_box_about() {
if ( is_page('about-us') ) : // Checks if the page's slug is 'about-us'
echo '<p style="position: absolute;
font-size: 25px;
top: 180px;
right: 100px;
padding: 10px;
background-color: #000000;
color: #FFFFFF;">This is the About Us page!</p>';
endif;
}
endif;
add_action( 'wp_footer', 'display_text_box_about' );
Now the function displays the text box only on the Page with the slug about-us
:
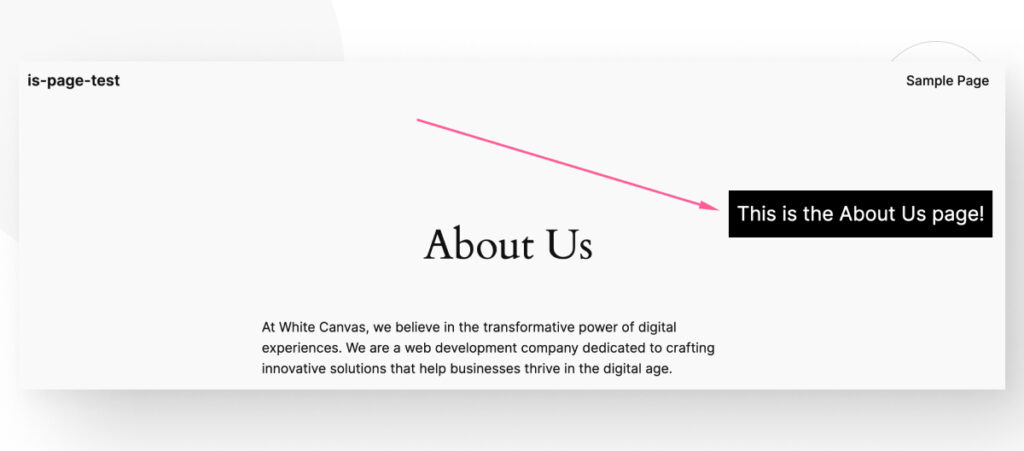
This is a very simple example, but it shows that you can execute code conditionally on WordPress pages using the is_page
function.
Bottom Line
The is_page
function determines whether the current page is a single Page, a WordPress content type typically used for static content like a Contact or About Us page.
Checking whether the current page is a single Page helps you run code conditionally, displaying unique widgets, sidebars, and other content elements only on single Pages. This is very useful for theme and plugin development.
If you found this post useful, read our blog and resources for more insights and guides!
Related Articles
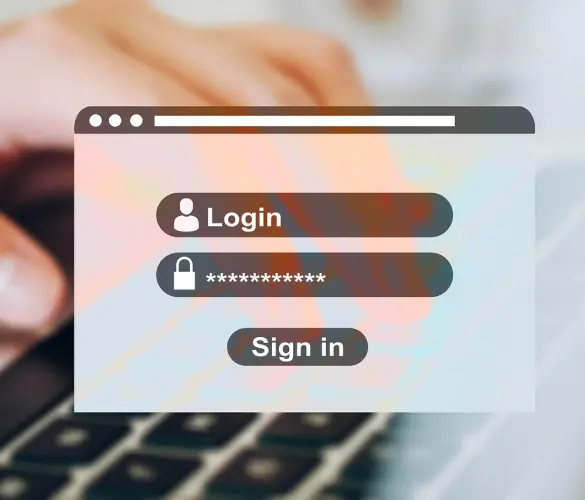
How to... / 5 min read
How to... / 5 min read
How to Make a Subscriber Into an Admin on WordPress?
While it's not the most common situation, at some point, you may find yourself needing to make a Subscriber into an Admin on your WordPress site. Maybe you need to…
Read More
How to... / 3 min read
How to... / 3 min read
How to Grant Secure Access to a Not Live WordPress Site During Development
When developing a WordPress site, you often need to collaborate with multiple developers and grant access to the website owner so they can review the progress themselves. However, granting access…
Read More
Industry Insights / 11 min read
Industry Insights / 11 min read
Do You Need a Web Developer to Build a WordPress Site?
If you’re building a WordPress site or considering building one, you wonder whether you need a web developer to create it or you can do it yourself. The answer can…
Read More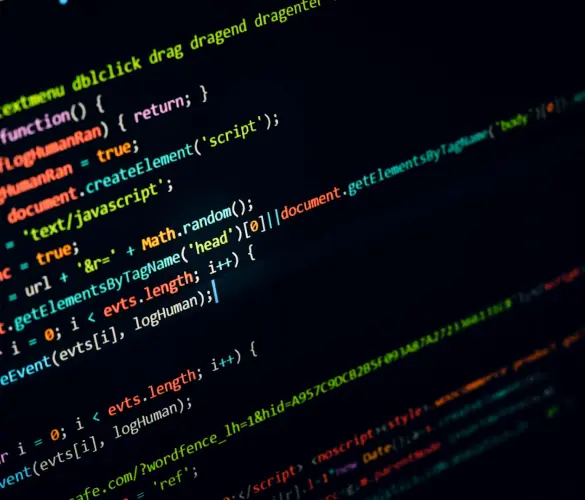
How to... / 6 min read
How to... / 6 min read
How to Change the Bottom Padding Dimensions on WordPress Blocks
If you're learning to modify your WordPress site's layouts and design, you may be wondering how to change the bottom padding dimensions of some of its elements. If that's the…
Read More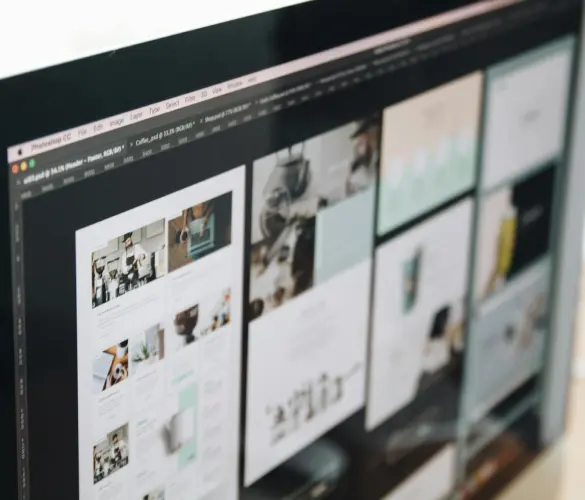
How to... / 10 min read
How to... / 10 min read
How to Disable the “Similar Posts” Section in WordPress Blogs
If you’re diving deeper into customizing your site, you may be wondering how to disable the “Similar Posts” section that appears on the bottom, sidebar, or footer of your WordPress…
Read More