- Resources
- JavaScript
- How to Use Swiper.js Callbacks for Better Slider Control in WordPress
JavaScript / 7 min read
How to Use Swiper.js Callbacks for Better Slider Control in WordPress
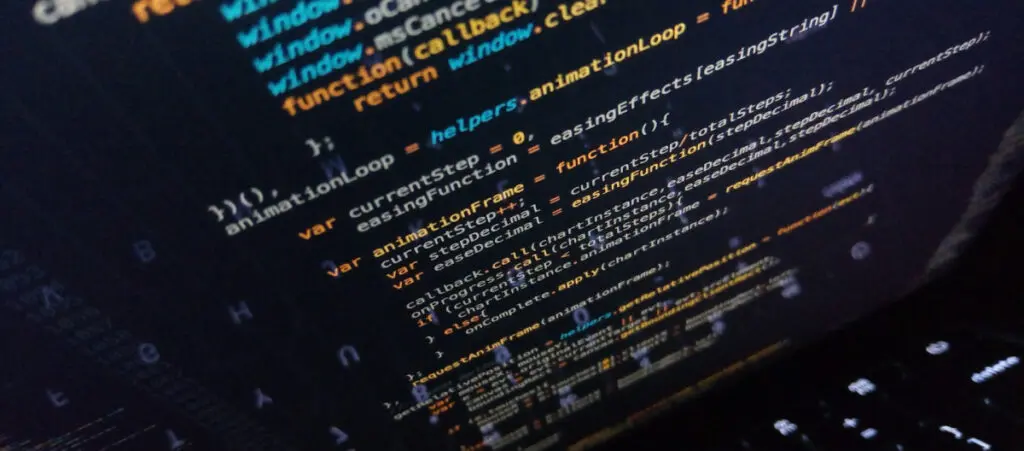
Sliders are essential web design elements we commonly find in galleries, product displays, and other website components. But how can you make sliders smarter, more dynamic, and responsive in WordPress development projects? For us, the answer has been Swiper.js, a free and open-source slider that works with React, Vue.js, and WebComponents.
Swiper.js provides a versatile touch slider WordPress developers can use for their websites. Through its robust API and callback system, you can integrate custom logic to control slider behavior and improve the user experience.
This post will explore how you can use Swiper.js callbacks in WordPress to create more responsive and engaging sliders.
Why Use Swiper.js in WordPress?
Swiper.js is a touch-enabled slider that is widely used in web and mobile applications. It offers flexibility, good performance, and it’s easy to integrate into WordPress, making it an excellent alternative if you’re looking for a slider solution for your site.
These are the main benefits you will enjoy if you decide to use Swiper.js for your WordPress site’s sliders:
- High performance. Swiper.js is optimized to ensure smooth transitions and interactions, even in server environments with limited resources or on slower devices.
- Extensive API. The Swiper.js allows you to control pagination, navigation, touch events, and more. It is the source of the slider’s flexibility.
- Easy integration with WordPress. Swiper.js integrates smoothly with WordPress themes and blocks, helping you easily deploy its features into existing templates or custom blocks.
The Importance of Swiper.js Callbacks in WordPress
Callbacks are functions executed at specific points during the slider’s life cycle. Callback functions are accessible by other functions and executed after the function that called it.
They provide the opportunity to inject custom functionality when certain actions occur, such as when a slide changes or a transition starts or ends.
These are the benefits of using Swiper.js callbacks in WordPress:
- Interactivity. Callbacks enable you to define what happens when a user interacts with the slider, such as triggering animations or preloading content when a slide transition begins.
- Content delivery. Using callbacks like slideChangeTransitionStart, you can dynamically load content, ensuring that resources are only requested when necessary, which improves both performance.
- Precision control. Callbacks allow for granular control over the slider, providing the ability to implement more complex user interactions, trigger AJAX requests, or synchronize other elements with slider actions.
2 Key Callbacks: slideChangeTransitionStart and slideChangeTransitionEnd
slideChangeTransitionStart
This callback is executed when the slider begins moving to the next or previous slide. It’s useful for resetting animations or loading content before the user sees the next slide, ensuring the next content is ready when the transition finishes. It uses 1 argument, swiper.
Here’s an example of how to use it:
swiper.on('slideChangeTransitionStart', function () {
resetSlideState(swiper.slides[swiper.activeIndex]);
});
This ensures that the new slide starts in a clean state, without any leftover animations or styles from previous slides.
slideChangeTransitionEnd
This callback executes after the slide has fully transitioned to the next or previous slide. It is often used to trigger events or animations that should happen once the new slide is fully visible. It uses 1 argument, swiper.
Here’s an example of how to use it:
swiper.on('slideChangeTransitionEnd', function () {
animateSlide(swiper.slides[swiper.activeIndex]);
});
With this callback, animations can start only after the slide is completely visible, providing a smooth user experience.
How to Implement Swiper.js in WordPress
Swiper.js can be seamlessly integrated into WordPress by enqueuing the necessary scripts and using callbacks to enhance user interaction. Here’s an example of how you can set up and enhance a Swiper slider with custom callbacks.
Enqueue Swiper.js in WordPress
Begin by adding the Swiper.js library and styles to your theme or plugin via wp_enqueue_script()
and wp_enqueue_style()
. Here’s the code you can use:
// Enqueue Swiper.js in WordPress
function enqueue_swiper_scripts() {
// Enqueue Swiper styles
wp_enqueue_style('swiper-style', '<https://unpkg.com/swiper/swiper-bundle.min.css>');
// Enqueue Swiper script
wp_enqueue_script('swiper-script', '<https://unpkg.com/swiper/swiper-bundle.min.js>', array(), null, true);
// Optionally, enqueue your custom JavaScript file that initializes Swiper
wp_enqueue_script('custom-swiper-init', get_template_directory_uri() . '/js/swiper-init.js', array('swiper-script'), null, true);
}
add_action('wp_enqueue_scripts', 'enqueue_swiper_scripts');
- swiper-style. Loads the Swiper CSS file.
- swiper-script. Loads the Swiper JavaScript from the CDN.
- custom-swiper-init. Optionally, load your custom JS file (swiper-init.js) where you’ll initialize Swiper and define its behavior.
Initialize Swiper.js
Now, in your custom JavaScript file (swiper-init.js
), you can initialize Swiper.js. Here’s how you can do it with callbacks:
// Custom JavaScript file (swiper-init.js)
document.addEventListener('DOMContentLoaded', function () {
const swiper = new Swiper('.swiper-container', {
slidesPerView: 1, // Only one slide visible at a time
navigation: {
nextEl: '.swiper-button-next', // Custom next button
prevEl: '.swiper-button-prev', // Custom previous button
},
on: {
// Triggered when the slide transition starts
slideChangeTransitionStart: function () {
resetSlideState(swiper.slides[swiper.activeIndex]);
},
// Triggered when the slide transition ends
slideChangeTransitionEnd: function () {
animateSlide(swiper.slides[swiper.activeIndex]);
}
}
});
});
Example HTML Structure for Swiper Slides
Here’s an example HTML structure that works with the above Swiper initialization:
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">
<div class="slide-content">
<h2>Slide 1 Content</h2>
<p>This is the first slide</p>
</div>
</div>
<div class="swiper-slide">
<div class="slide-content">
<h2>Slide 2 Content</h2>
<p>This is the second slide</p>
</div>
</div>
<!-- Additional slides... -->
</div>
<!-- Navigation buttons -->
<div class="swiper-button-next"></div>
<div class="swiper-button-prev"></div>
</div>
Best Practices for Swiper.js Callbacks
To get the most out of Swiper.js in WordPress, it’s important to follow some best practices when working with callbacks.
Make Your Code Modular
Break down complex logic into smaller, reusable functions. This keeps the callback functions clean and ensures your codebase is easier to manage and debug. For instance:
function resetSlideState(slide) {
// Logic to reset the slide state
const content = slide.querySelector('.slide-content');
content.style.opacity = '0'; // Reset opacity
content.style.transform = 'translateY(20px)'; // Reset position
}
function animateSlide(slide) {
// Logic to animate the slide
const content = slide.querySelector('.slide-content');
content.style.transition = 'opacity 0.5s ease, transform 0.5s ease';
content.style.opacity = '1'; // Fade-in animation
content.style.transform = 'translateY(0)'; // Slide-up animation
}
swiper.on('slideChangeTransitionStart', () => {
resetSlideState(swiper.slides[swiper.activeIndex]);
});
swiper.on('slideChangeTransitionEnd', () => {
animateSlide(swiper.slides[swiper.activeIndex]);
});
Optimize for Performance
Avoid overloading your callbacks with too much logic. Use them to trigger essential functions, and ensure only necessary content is loaded during transitions to maintain performance, especially on mobile devices.
Test Across Devices
Ensure your slider works smoothly on different screen sizes and devices. Thorough testing ensures consistency and an optimal user experience, especially since mobile devices handle touch and swiping gestures differently.
Stay Updated
Swiper.js is frequently updated with new features and performance enhancements. Make sure you’re using the latest version to benefit from bug fixes and improved functionality.
Benefits of Leveraging Swiper.js Callbacks
By leveraging Swiper.js callbacks, developers gain powerful tools to create engaging and high-performing sliders in WordPress. Here are some key benefits:
- Improved User Experience: With callbacks, developers can control precisely when content is displayed or hidden, providing smoother transitions and a more engaging experience for users.
- Performance Optimization: Load content dynamically during slide transitions, reducing the initial page load and improving the performance of your site, particularly on slower connections or mobile devices.
- Greater Control: Swiper.js callbacks offer fine-grained control over the behavior of your slider, allowing you to tailor it to specific project requirements, whether it’s for custom animations, dynamic content loading, or interactive elements.
Swiper.js Callbacks Make it Easier to Manage Sliders in WordPress
Swiper.js provides WordPress developers with a robust tool for building highly interactive and flexible sliders. Its features will help you improve the user experience, enhance performance, and gain greater control over how your slides behave.
Hopefully, this post served as a good introduction to using Swiper.js in WordPress and you can apply what you learned here on your site.
If you found this post useful, read our blog and developer resources for more insights and guides!