- Blogs
- Development
- WordPress get_post_type function: what it is and how to use it
Development / 9 min read
WordPress get_post_type function: what it is and how to use it
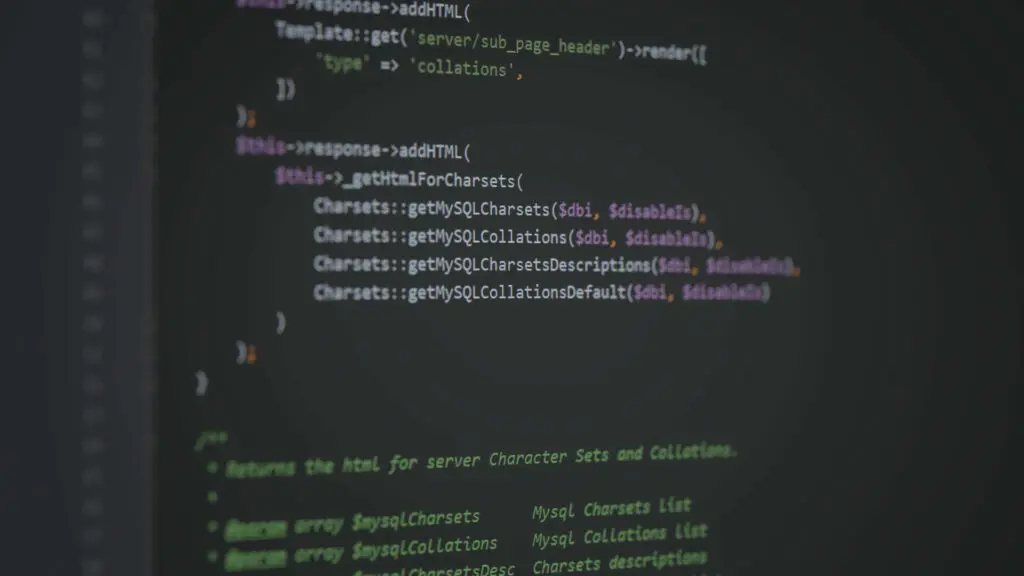
The WordPress get_post_type
function is a built-in function that identifies one of the fundamental aspects of the platform’s content management features: the post type.
In this article, we’ll explain post types, how the get_post_type
WordPress function helps you identify them, and how you can use this function to perform selective actions on specific post types.
Key Takeaways
- Post types are the different kinds of content WordPress manages.
- get_post_type is a built-in WordPress function that identifies the type of a specific post or the current post in the WordPress loop.
- get_post_type helps you apply styles and web elements or perform actions only when you’re on specific post types.
What Are Post Types in WordPress?
Since the get_post_type
function deals with post types, it’s essential to understand what WordPress post types are, especially if you’re new to the platform. If you’re already familiar with post types, skip to the section “What Is the get_post_type Function?”
A post type is a specific type of content within the WordPress CMS. Originally, WordPress was mostly a blogging platform, so every piece of content was simply a “post.” However, as WordPress expanded its content management features, new types of content appeared.
Post types expand WordPress’s reach beyond blogging, allowing sites of all types to publish meaningful content to their audience in a structured way.
Default and Custom Post Types
Post types can be either default post types or custom post types. The following are the post types included in your WordPress installation by default, stored in your database under the wp_posts
table.
Default Post Types
- Posts. The post type most commonly used for blogging. Your blog archive will typically display posts in reverse chronological order (newest to oldest), making them ideal for content you update regularly, such as news articles.
- Pages. Pages show “static” content. Static content is content that doesn’t change frequently. Examples include informational web pages such as “About Us” and “‘Privacy Policy.” Pages are not listed chronologically and don’t use tags or categories like posts often do.
- Attachments. Any piece of media uploaded to your site. It includes images, videos, audio files, or PDF documents.
- Revisions. A revision is a version of a specific piece of content. Each time you make changes and save them in a post or page, WordPress automatically creates a revision, allowing you to view and revert to previous versions of your content.
- Navigation Menus (“Menus”). Menus are lists of links that help users navigate your website more efficiently. This post type allows you to customize lists of links to various pages and posts on your site.
- Custom CSS. This post type is theme-specific. Each time you use the Customizer (Appearance > Customize > Additional CSS) to add custom CSS styles, WordPress saves these changes and associates them with the theme you just customized.
- Changesets. Changesets are like revisions for the Customizer mentioned above. WordPress saves each change into a changeset similar to a draft.
Custom Post Types
In addition to using the default post types, you can also create your own post types with the custom post types feature. Custom post types are excellent for creating templates that don’t fit into default post types and require a personalized structure. Examples include case studies, portfolios, team member profiles, and event pages.
You can create custom post types using the native function register_post_type
, but we recommend using the Advanced Custom Fields plugin instead. It makes the process easier and ensures the custom posts remain after switching themes.
What Is the WordPress get_post_type Function?
Now we’ve explored post types, you can better understand what the get_post_type
does.
The get_post_type
function retrieves a text string with the post type of the current post or any specific post you want. It queries the WordPress database to determine the type of a given post.
get_post_type
can be very useful in multiple scenarios, such as applying specific layouts or styles based on the post type or displaying different sidebars or custom fields for different post types.
How Does the WordPress get_post_type Function Work?
The basic syntax of the get_post_type
function is:
get_post_type( $post = null );
The only parameter is $post
, and it’s optional.
The value of $post
can be a specific post ID (an integer), a WP_Post
object (post object), or left blank. In case a value isn’t specified, it automatically defaults to the null
value and retrieves information from the current post in the WordPress loop.
If the parameter’s value is valid, it returns the type of that post. If the value isn’t valid, it returns false
, indicating the post type couldn’t be determined.
Breaking Down WordPress’s get_post_type Function
For a deeper explanation, we can find the definition of get_post_type
in the WordPress core. More specifically, it’s in the post.php
file inside the wp-includes
folder:
<?php
/**
* Retrieves the post type of the current post or of a given post.
*
* @since 2.1.0
*
* @param int|WP_Post|null $post Optional. Post ID or post object. Default is global $post.
* @return string|false Post type on success, false on failure.
*/
function get_post_type( $post = null ) {
$post = get_post( $post );
if ( $post ) {
return $post->post_type;
}
return false;
}
?>
Since the function is pretty short, we can break down what each line of code does.
The 1st Line
function get_post_type( $post = null ) {
The first line declares the get_post_type
function and its one parameter, $post = null.
The 2nd Line
$post = get_post( $post );
The second line is when the function body starts. It attempts to get the post using $post = get_post( $post );
. get_post
is a built-in WordPress function that retrieves a post based on a specific post ID or post object.
If you pass it a post ID (an integer), it returns the corresponding post object, but if you pass it a post object, it simply returns that object back. Finally, if you pass null
or no argument, it attempts to retrieve the current global post object, which is the current post in the WordPress loop.
This second line ensures the $post
variable is always a post object. Regardless of the initial input, it will always return a post object. This is important because the rest of the function operates on a post object.
By ensuring the $post
variable is always a post object (or null
if the post doesn’t exist or isn’t specified), the function can always reliably access the post_type
property of the WP_Post
object later on.
The 3rd Line
if ( $post ) {
This line checks whether the variable $post
is true
or false
. If this conditional returns true
, the post exists, and the program moves to the following line. If it returns false
, it means no post was found, either because there was no current post in the loop or the provided post ID was invalid.
The 4th Line
return $post->post_type;
If the previous line found a post, this line accesses the post_type
property of the post object. This property contains the type of the post (post, page, attachment, etc.) and the function returns it as a string.
The Last Few Lines
}
return false;
}
The fifth line closes the brackets opened by the conditional ( if ( $post ) {
).
return false;
means the function returns a Boolean false
if no post was found. A false
indicates the post type could not be determined. This can happen because there is no current post on the loop or the specified post does not exist.
Finally, the last line ( }
) closes the function.
How To Use get_post_type In WordPress?
Now that you know how the get_post_type
function works on a deeper level, it’s time to use it. The following snippet is a simple example of how to use the function to check the current post’s type to display the message “This is a post!” The idea is to only display the message if the post type is post. Remember that post
is the post type for blog entries.
You can place the snippet in the single.php
file, which is the script WordPress uses to render individual posts.
Here’s the code:
<?php
if (have_posts()) : while (have_posts()) : the_post();
// Get the post type for the current post
$post_type = get_post_type();
if ($post_type == 'post') {
echo "This is a post!";
}
// Rest of the code that shows the post's content
endwhile; endif;
?>
In the first list line, we check whether there are any posts to display with if (have_posts()
. This is a standard WordPress loop control structure.
Then we start the loop with while (have_posts())
. If there are posts available, the function iterates through them. the_post()
sets up the post data, preparing it for retrieval and display.
In the second line, $post_type = get_post_type();
calls the get_post_type function to identify the post type of the current post in the loop. We store the retrieved post type in the variable $post_type.
The third line starts the conditional check for the post type. if ($post_type == 'post') {
checks if the post type of the current post is post
. If the post type is indeed post
, it issues an echo statement to display the message This is a post!
.
Finally, endwhile; endif;
ends the loop and ends the if
statement that began the loop.
This is a very simple example, but it gives you an idea of how to use the get_post_type
function in your WordPress development project.
Final Thoughts on the get_post_type Function
Post types are the different types of content WordPress manages, including images, blog posts, and custom types you can create to fit your website’s content needs. The get_post_type
function determines the type of a given post in WordPress.
get_post_type
can be very useful for applying specific layouts or styles based on the post type or performing specific actions, such as displaying a message to identify the current post’s type. Hopefully, you now better understand how it works and how you can use it in your projects.
If you found this post useful, read our blog for more WordPress insights and guides!
Related Articles
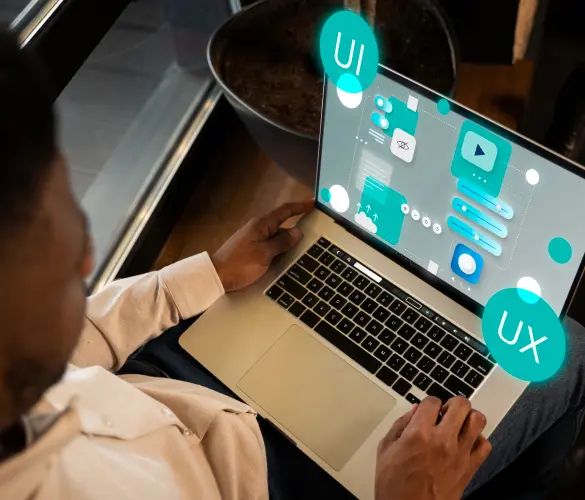
Development / 6 min read
Development / 6 min read
The Benefits of the Full Site Editor for WordPress Users
Full Site Editor (FSE) is a WordPress feature that allows any user to modify their entire site through the familiar interface of the Gutenberg editor. This makes FSE a great…
Read More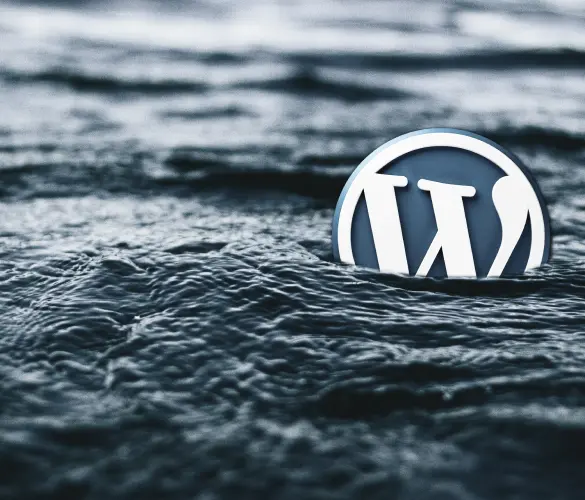
Development / 14 min read
Development / 14 min read
Is WordPress Dying in 2024?
The question, "Is WordPress dying?" seems to be as old as WordPress itself, but is there any truth to it? If you're considering whether you should use WordPress in 2024…
Read More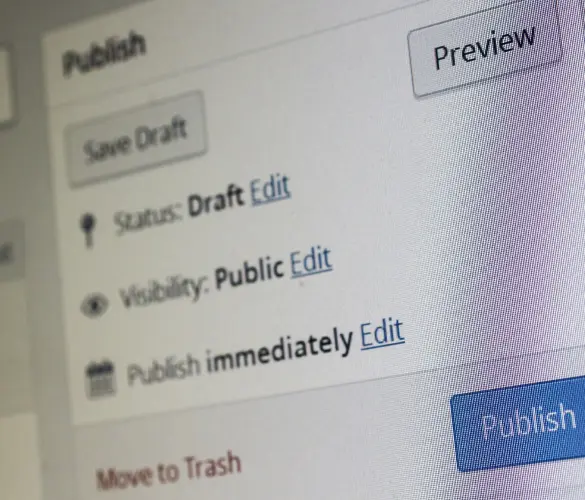
Development / 2 min read
Development / 2 min read
How to Publish Draft Pages in WordPress?
If you’re learning how to use WordPress and wondering how to publish your draft pages or posts, the process is actually really simple, and this article will show you how…
Read More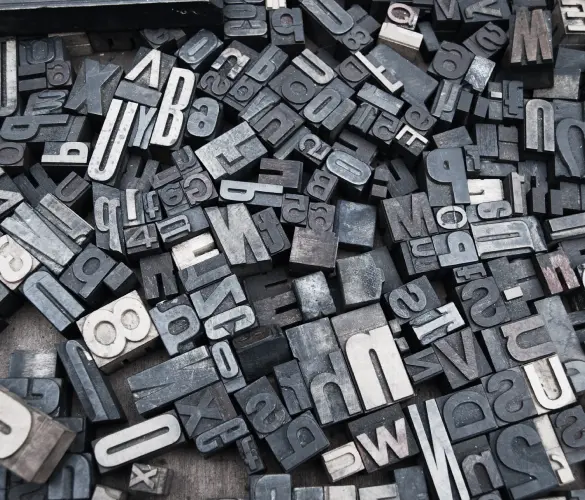
Development / 4 min read
Development / 4 min read
How to Change the Font Color in WordPress: 4 Easy Methods
Are you wondering how to change the font color in your WordPress site? Many people do when looking for a font color that balances readability with brand identity. In this…
Read More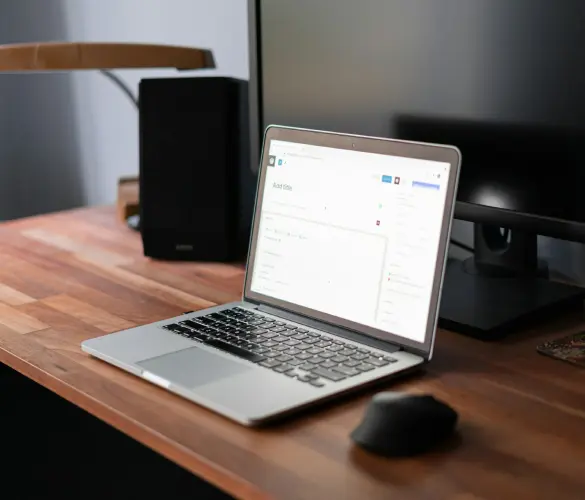
Development / 9 min read
Development / 9 min read
How to Fix the WordPress “Cookie Check Failed” Error
The “cookie check failed” error is a message that appears on some WordPress sites, preventing users from accessing some or all content and admins from managing the website. Let’s explore…
Read More