- Blogs
- Development
- WordPress is_front_page tag: what it is and how to use it
Development / 8 min read
WordPress is_front_page tag: what it is and how to use it
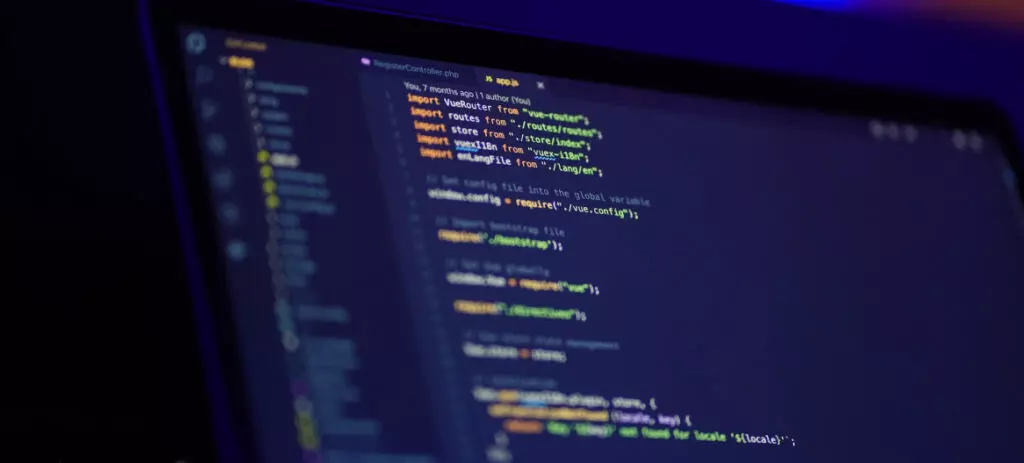
WordPress has many functions that allow you to develop context-aware and flexible plugins and themes. is_front_page
is one of those functions, helping you determine whether the user is on the WordPress front page, a check that may come in handy depending on your goals.
Let’s explore the WordPress is_front_page
function, how it works, how it relates to the similar function is_home
, and how your site’s settings may modify its behavior.
Key takeaways
- is_front_page is a WordPress conditional tag that determines whether the user is in the site’s root URL (the site’s front page).
- is_home is a conditional tag that determines whether the user is on the page displaying the blog posts index (your latest blog posts).
- The site configurations in Settings > Reading alter how is_front_page and
is_home
behave when you use them on your front page and homepage. - When developing themes and plugins, consider all possible results that is_front_page and is_home can return based on the site’s settings.
What is the is_front_page function in WordPress?
is_front_page
is a WordPress conditional tag that theme and plugin developers use to determine whether users are currently on the WordPress front page. The front page is the site’s main landing page. That is, the page you arrive at when you access any WordPress website’s root URL. For example, our site’s front page is https://wcanvas.com/
.
The is_front_page
function helps developers determine whether the user is currently on the front page.
Before moving on, it’s important that you don’t confuse the front page with the homepage, as these terms may seem interchangeable, but, in reality, they’re different. The homepage is the page that lists the latest blog posts in reverse chronological order (newest to oldest). In other words, you can think of the homepage as the blog homepage or the blog posts index.
As you may have noticed, the blog posts index is what WordPress displays by default when you start a new WordPress website.
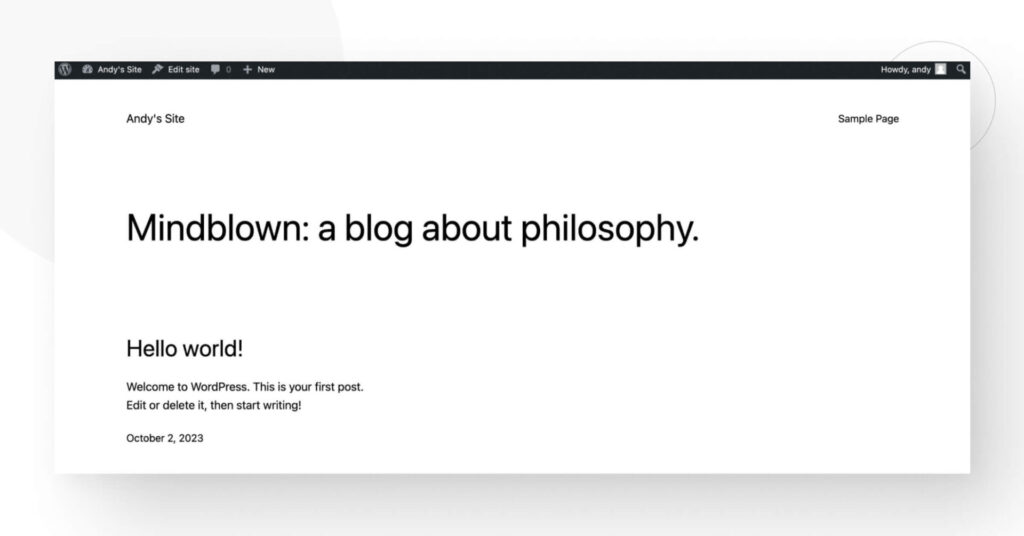
So, by default, the WordPress front page and homepage are indeed the same. But you can change this behavior by navigating to Settings > Reading from the WordPress dashboard.
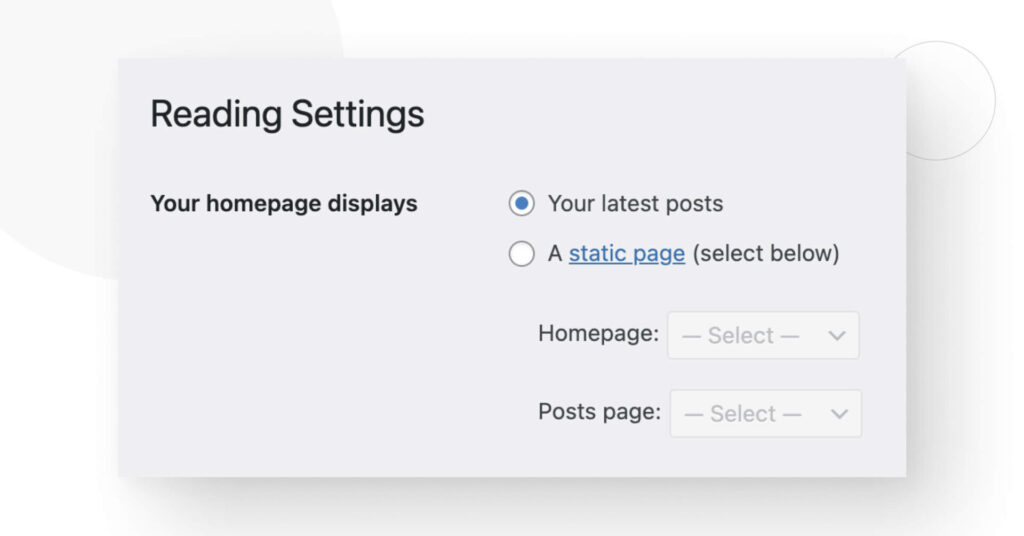
As you can see, WordPress makes your front page (your root URL) display your latest posts by default. By choosing A static page (select below), you can select one of your pages to display in your site’s main URL from a drop-down menu.
This selected page is known as the static front page. The contents of this static front page can still be dynamic, but it’s different from the blog posts index.
Front page and homepage summary
To summarize:
- Your front page is the page that displays when someone visits your site’s root URL. On our site, it’s https://wcanvas.com/.
- Your homepage is the page that displays your latest blog posts in reverse chronological order. On our site, it’s https://wcanvas.com/blogs/.
- Depending on your settings, these pages can be different or the same, but they’re still two separate concepts.
With that cleared up, let’s learn how to use is_front_page
and a similar function, is_home
.
Using is_front_page and is_home in WordPress
is_front_page
and is_home
are conditional tags. Conditional tags are PHP functions that allow you to check whether the current post, page, or request matches a specific condition.
Developers use these tags mostly in theme and plugin development to selectively display or hide content, apply specific CSS styles, execute more PHP code, and more. If the statement evaluated by the tag returns true
, the style is applied or the code executes.
Keep in mind that you can only use these tags when WordPress is loaded. That means you can use them on template files for classic themes and action hooks, but they won’t work if you just paste them into functions.php
.
Let’s see how to use these tags in your theme or plugin.
Use is_front_page to display a message in the static front page
The following code checks whether the user is currently on the front page (static or otherwise) and displays a message if they are.
<?php
if (is_front_page()) {
// This code only executes on the static front page
echo "<h2>Welcome to our front page!<h2>";
} else {
echo "<h2>You're not on our front page anymore.<h2>";
}
?>
With this snippet, if is_front_page
returns true
, then your front page will display a message. If it returns false
(when the user is on any other page other than the front page), a different message displays.
Displaying messages like this is unwieldy and not recommended, but it’s an example of what you can do with this conditional tag.
Use is_home to display a message in the page for the latest blogs
is_home
plays a similar role to is_front_page
but returns true
when the user is in the blog posts index. Here’s an example of how you can use it:
<?php
if (is_home()) {
// This code will execute if you are on the homepage when it displays blog posts.
echo "<h2>Welcome to our blog's homepage!<h2>";
} else {
// This code will execute for other pages on your site.
echo "<h2>You're not on our blog's homepage anymore.<h2>";
}
?>
In this snippet, if you are on the homepage (is_home
returns true
), you’ll see the “Welcome to our blog’s homepage!” message. If you are on any other page of your website, is_home returns false
, and you’ll see the “You’re not on our blog’s homepage anymore” message.
How WordPress settings change is_front_page and is_home’s behavior
As mentioned before, the configurations you select in Settings > Reading influence what users see when they visit your root URL. The default configuration is Your latest posts, making your homepage and front page the same. If you select A static page (select below), your front page and homepage stop being the same.
These changes influence whether is_home
and is_front_page
will return true
or false
. This is crucial because you should always know what the functions will return when coding themes or plugins to prevent unexpected behavior. Here’s a quick guide to how the Reading settings alter the results of these two functions.
Default settings
When you leave the Reading settings as Your latest posts, your front page will display your latest posts. Here’s what each function returns when you test them on your front page:
is_home
returnstrue
.is_front_page
returnstrue
.
is_front_page
always returns true
if you’re on the WordPress front page, whether it is the default front page or a custom one.
You’re using a static front page
When the Reading settings are set to A static page (select below), your front page will display the custom page you selected in the drop-down menu. Here’s what each function returns when you test them on your front page:
is_home
returnsfalse
.is_front_page
returnstrue
.
You’re using a custom homepage to list your blogs
When the Reading settings are set to A static page (select below), your homepage will display the custom page you selected in the drop-down menu. Here’s what each function returns when you test them on your custom homepage:
is_home
returnstrue
.is_front_page
returnsfalse
.
How to take the Reading settings into account when coding themes and plugins
Because some of your users will change the Reading settings, your theme or plugin must consider all possible scenarios. Here’s a proposed logic you can use to take every combination into account.
<?php
if ( is_front_page() && is_home() ) {
// Execute this code when the homepage and the front page display recent posts (is_front_page and is_home return True)
} elseif ( is_front_page() ) {
// Execute this code when front page and homepage are different, and the user is in your custom static front page
} elseif ( is_home() ) {
// Execute this code when front page and homepage are different, and the user is in the recent posts page
} else {
// Execute this code when the user is in any other page
}
?>
Feel free to tweak this snippet and make it more specific to your needs!
Bottom line
is_front_page
is a WordPress conditional tag that determines whether the user is in the site’s root URL (the site’s front page). is_home
is a conditional tag that determines whether the user is on the page displaying your latest blog posts (the blog posts index).
By default, the front page and homepage are the same, but you can change this behavior by going to Settings > Reading from your WordPress dashboard. Depending on these settings, is_home
and is_front_page
will return true
or false
when tested in your root URL.
We provided a list of possible results depending on your settings and a template logic you can use on your theme or plugin to take each one into account. Tweak it as you see fit.
If you found this post useful, read our blog for more WordPress insights and guides!
Related Articles
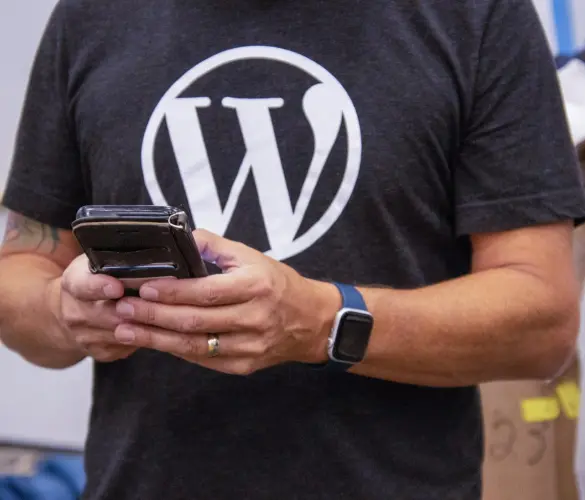
Development / 11 min read
Development / 11 min read
WordPress Doesn’t Display Correctly on Mobile: 5 Solutions
Sometimes, your WordPress site doesn't display correctly on mobile devices despite working just fine on desktop. This can be frustrating and hard to troubleshoot because there are so many reasons…
Read More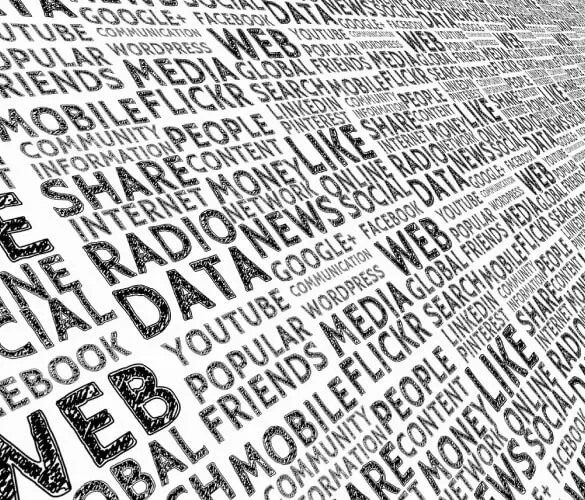
Development / 8 min read
Development / 8 min read
Why Is WordPress Missing the Color and Underline Font Options?
WordPress is the most popular CMS in the world, and many use it because its editor is intuitive and easy to learn. But sometimes, site owners or developers find the…
Read More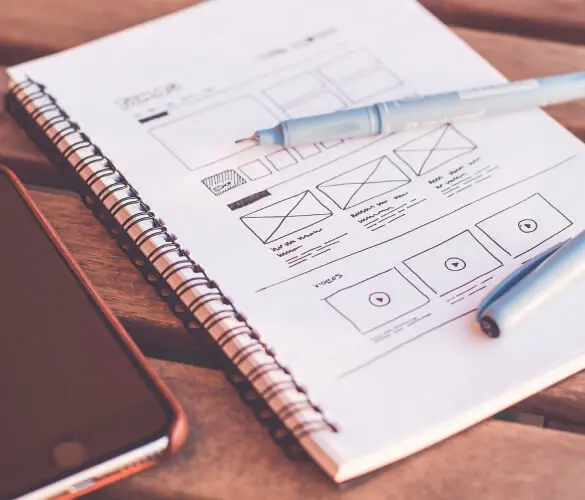
Development / 5 min read
Development / 5 min read
How to Convert a Container-Based Layout to a Column-Based Layout in WordPress
Columns and containers are some of WordPress's most popular layout styles. Depending on the project's specifications and your personal preferences as a designer or developer, you may need to convert…
Read More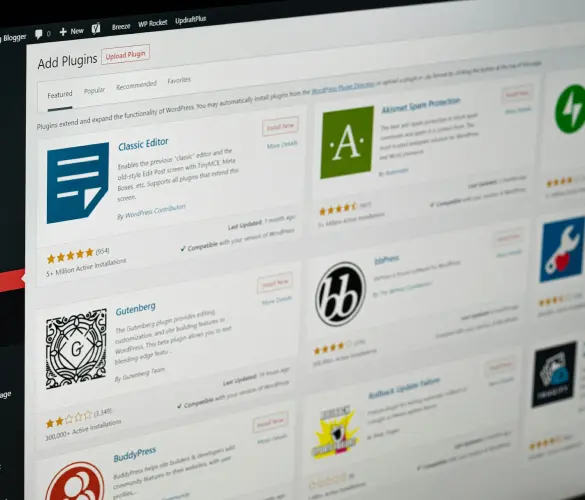
Development / 5 min read
Development / 5 min read
Why Is Your Animated GIF Not Working in WordPress? 3 Solutions
It can be very frustrating to upload a GIF to add life to your post, only to find out that your animated GIF is not working in WordPress. So, why…
Read More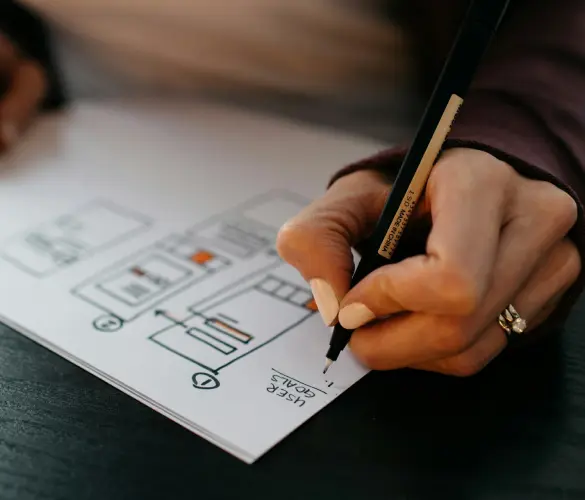
Development / 9 min read
Development / 9 min read
What Are WordPress Maintenance Packages: Cost and Benefits
WordPress requires ongoing maintenance to keep your themes and plugins updated, your site safe from security vulnerabilities, and your performance and speed high to offer a better user experience. WordPress…
Read More