- Blogs
- WordPress 101
- WordPress get_site_url function: What it Is and How to Use it
WordPress 101 / 7 min read
WordPress get_site_url function: What it Is and How to Use it
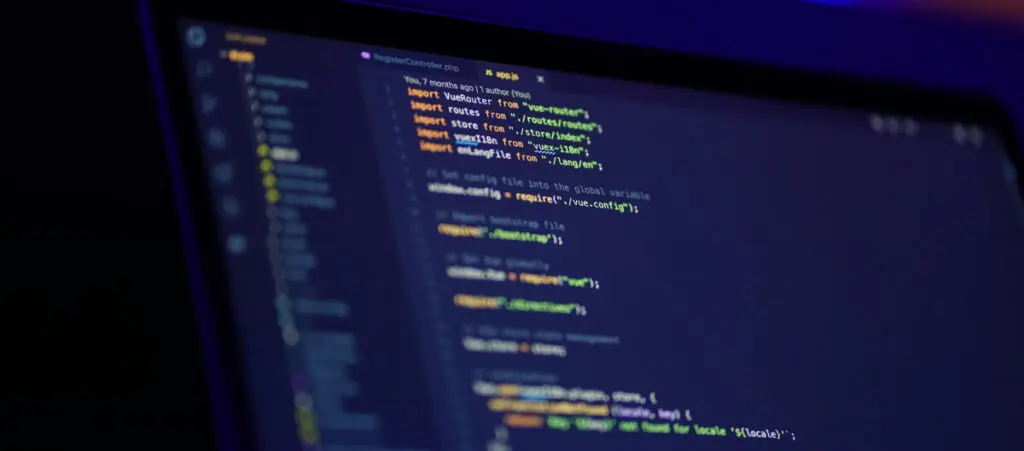
The get_site_url WordPress function allows developers to retrieve the URL of the current site or any site in a multisite network. Let’s explore how the function works and provide examples of how to use it in your site.
Key Takeaways
- get_site_url retrieves the URL of the current WordPress site it’s being used on and any site in a multisite network.
- You can use get_site_url in custom plugins or edit your theme’s functions.php file.
What is get_site_url in WordPress?
The get_site_url
function retrieves the URL for the current site. get_site_url
uses the get_option function, among others, to retrieve and return a string that represents the siteurl
option found in the wp_options
database table.
In individual installations, get_site_url
returns the URL of the current site. In multisite installations, get_site_url
can return the URL of the current site or return the URL for a specified site in the network.
How Does the get_site_url Function in WordPress?
get_site_url
’s definition is in the link-template.php
file, which is in the wp-includes
core folder:
function get_site_url( $blog_id = null, $path = '', $scheme = null ) {
if ( empty( $blog_id ) || ! is_multisite() ) {
$url = get_option( 'siteurl' );
} else {
switch_to_blog( $blog_id );
$url = get_option( 'siteurl' );
restore_current_blog();
}
$url = set_url_scheme( $url, $scheme );
if ( $path && is_string( $path ) ) {
$url .= '/' . ltrim( $path, '/' );
}
return apply_filters( 'site_url', $url, $path, $scheme, $blog_id );
}
get_site_url
has 3 parameters:
- $blog_id: The ID of the blog/site (default is null).
- $path: The path to append to the site URL (default is an empty string).
- $scheme: The URL scheme (http, https, etc.). Default is null.
With that in mind, let’s break down what each line means.
Step 1: Check Whether Blog ID is Set or Multisite
The first block of code determines whether the developer provided a $blog_id
or if the current WordPress site is a multisite installation:
function get_site_url( $blog_id = null, $path = '', $scheme = null ) {
if ( empty( $blog_id ) || ! is_multisite() ) {
$url = get_option( 'siteurl' );
} else {
switch_to_blog( $blog_id );
$url = get_option( 'siteurl' );
restore_current_blog();
}
If $blog_id
is empty or the site is not part of a multisite network, the function get_option
retrieves the current site’s siteurl
option.
If $blog_id
is set and the site is part of a multisite network, it switches to the specified blog using switch_to_blog( $blog_id)
. switch_to_blog
allows developers to pull posts and other information from other sites by switching the current context.
Using switch_to_blog
, get_site_url
retrieves the siteurl
option for that blog and then switches back to the current blog’s context using restore_current_blog
.
Step 2: Set the URL Scheme
The following line sets the URL scheme (http
or https
) based on the $scheme
parameter. If $scheme
is null
, the function uses the site’s current scheme.
$url = set_url_scheme( $url, $scheme );
Step 3: Append Path
If the $path parameter is provided and is a string, the function appends its value to the URL. ltrim( $path, '/' )
removes any leading slashes from the path to avoid double slashes in the URL.
if ( $path && is_string( $path ) ) {
$url .= '/' . ltrim( $path, '/' );
}
Step 4: Apply Filters and Return URL
The apply_filters
function uses 'site_url'
as the hook, $url
as the value to filter, and $path
, $scheme
, and $blog_ID
to the filter.
return apply_filters( 'site_url', $url, $path, $scheme, $blog_id );
Finally, the function returns the modified URL.
How to use the get_site_url Function in WordPress?
Some potential uses for get_site_url
include:
- Generating links to one of your subsites in a multisite network.
- Enforcing HTTPS on a link.
- Generating dynamic links based on specific conditions or user interactions.
- Generating API endpoints.
Let’s explore a couple of examples of how to use get_site_url
on your WordPress site.
Using get_site_url on Individual Installations
Let’s use get_site_url
to display a text box on your blog posts. This feature will use the get_site_url
function to capture your site’s URL in a variable and display it as a black text box with white text on every single page on your site.
Go to your theme’s functions.php
file to add the code. We’re using the Twenty Twenty-Four theme, so our functions.php
file is located in public > wp-content > themes > twentytwentyfour.
Add the following code:
if ( ! function_exists ( 'display_text_box' ) ) :
function display_text_box() {
if ( is_single() ) : // Checks if the page is a single page, such as a blog post
// Capture the site's URL into a variable
$url = get_site_url();
// Display the site's URL in a text box that appears over every single page
echo '<p style="position: absolute;
font-size: 25px;
top: 100px;
right: 100px;
padding: 10px;
background-color: #000000;
color: #FFFFFF;">This site\'s URL is: ' . $url . '</p>';
endif;
}
endif;
add_action( 'wp_footer', 'display_text_box' );
Here’s what this function looks like on your live site:
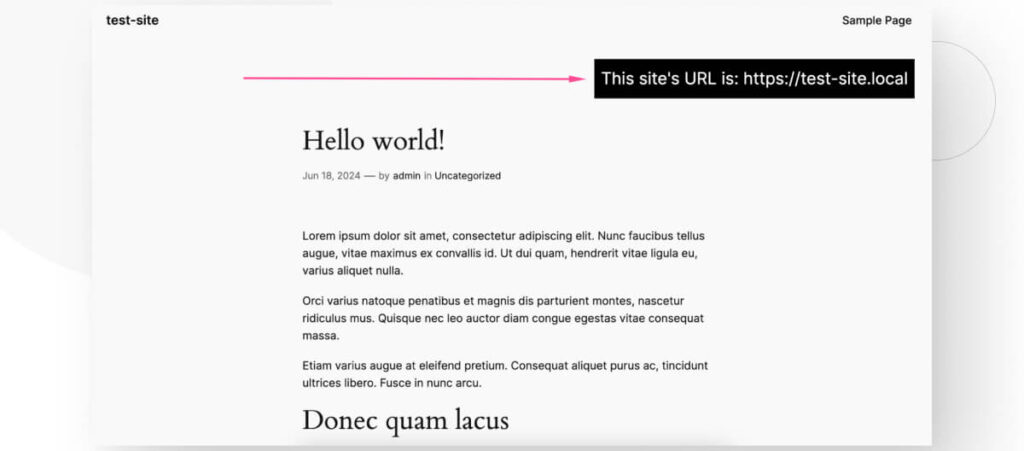
The text reads, This site's URL is: https://test-site.local
. This example was created on a local site using Local by Flywheel, if you try this on a live site, the URL would be https://test-site.com
.
To confirm whether the URL on display is correct, go to Settings > General.
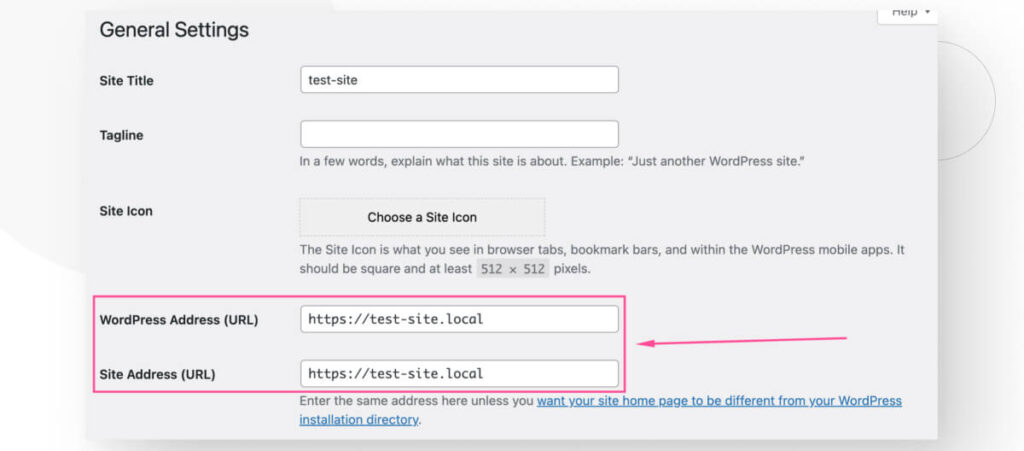
The URLs in Site Address (URL) and WordPress Address (URL) text boxes should be the same as the one displayed in our function’s text box since, most of the time, your Site and WordPress addresses are the same.
In some cases, such as when you install WordPress on a subdirectory, the Site and WordPress address will differ because they correspond to different keys in the wp_options
table. The Site address corresponds to the siteurl
key, and the WordPress address corresponds to the home
key.
In cases where your WordPress installation is in a subdirectory, the home
and siteurl
keys will have different values since the installation is not on your website’s root directory.
Using get_site_url on Multisite Installations
You can also use get_site_url
to display the URLs of multiple sites from the same multisite network by using the $blog_id
parameter.
For this example, we have a multisite network with three subdomains: Subdomain 1, Subdomain 2, and Subdomain 3.
We’ll add a slightly modified version of the code above in the main site’s functions.php
file to display the URLs of the main site and all subdomains:
if ( ! function_exists ( 'display_text_box' ) ) :
function display_text_box() {
if ( is_single() ) : // Checks if the page is a single page, such as a blog post
// Capture the site's URL into a variable
$url_subdomain_main_site = get_site_url(1);
$url_subdomain_one = get_site_url(2);
$url_subdomain_two = get_site_url(3);
$url_subdomain_three = get_site_url(4);
// Display the site's URL in a text box that appears over every single page
echo '<p style="position: absolute;
font-size: 18px;
top: 100px;
right: 100px;
padding: 10px;
background-color: #000000;
color: #FFFFFF;">The main site\'s URL is: ' . $url_subdomain_main_site .
'<br>' . 'Subdomain 1\'s URL is: ' . $url_subdomain_one .
'<br>' . 'Subdomain 2\'s URL is: ' . $url_subdomain_two .
'<br>' . 'Subdomain 3\'s URL is: ' . $url_subdomain_three .'</p>';
endif;
}
endif;
add_action( 'wp_footer', 'display_text_box' );
Here’s what this function looks like on your live site:
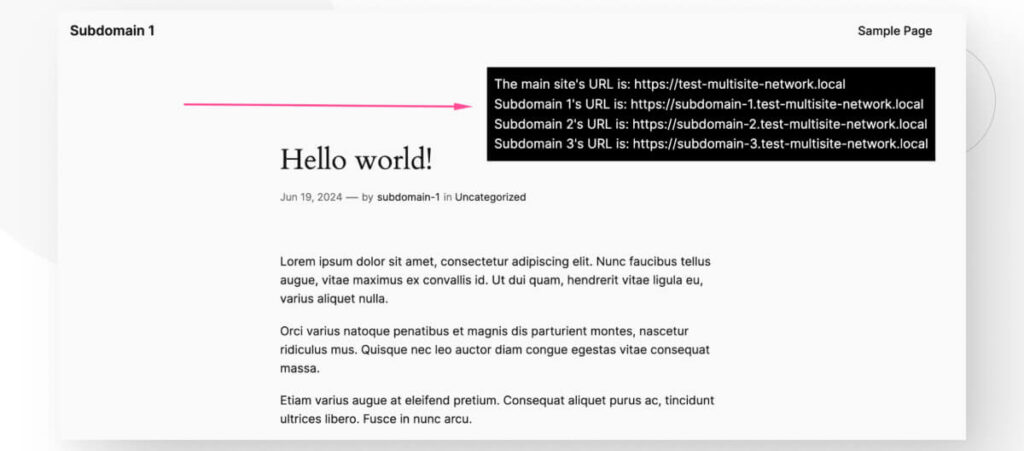
The text box reads:
The main site’s URL is: https://test-multisite-network.local
Subdomain 1’s URL is: https://subdomain-1.test-multisite-network.local
Subdomain 2’s URL is: https://subdomain-2.test-multisite-network.local
Subdomain 3’s URL is: https://subdomain-3.test-multisite-network.local
In a multisite installation, site ID 1
goes to the first (main) site created, and all following subsites receive an ID corresponding to the order in which they were created.
Get_site_url Helps You Capture and Display Your WordPress Address
The get_site_url
function retrieves the URL of your current site or any of the sites in your multisite network. It can be useful for generating links, enforcing HTTPS on a link, and generating dynamic links based on specific conditions or user interactions.
Now that you know how the function works, start using it to customize your site’s functionality.
If you found this post useful, read our blog and resources for more insights and guides!
Related Articles
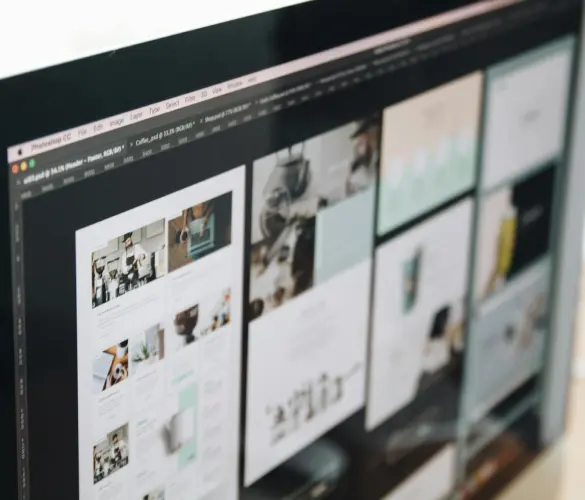
How to... / 10 min read
How to... / 10 min read
How to Disable the “Similar Posts” Section in WordPress Blogs
If you’re diving deeper into customizing your site, you may be wondering how to disable the “Similar Posts” section that appears on the bottom, sidebar, or footer of your WordPress…
Read More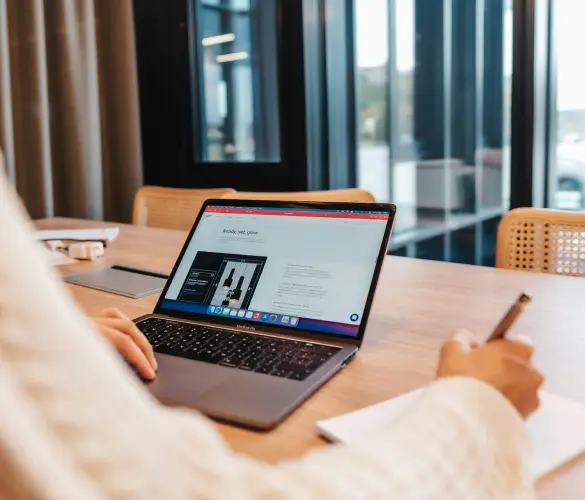
How to... / 8 min read
How to... / 8 min read
How to Change the Width of a Blog Post on WordPress (3 Methods)
If you're diving deep into WordPress web design, you'll eventually need to learn how to change the width of a blog on your WordPress site. It can improve readability or…
Read More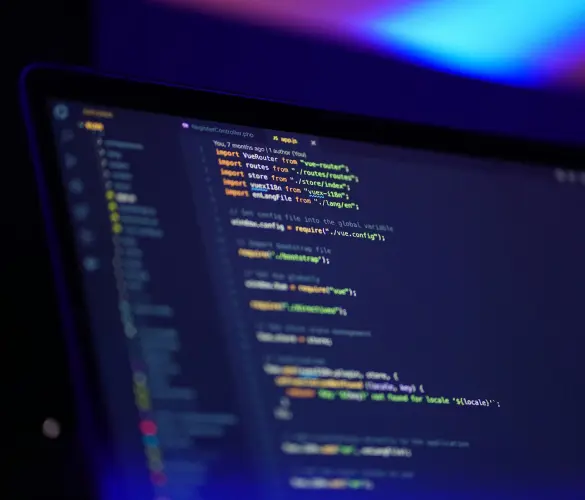
How to... / 3 min read
How to... / 3 min read
How to Hook Code Output After Content on WordPress?
When you're customizing your WordPress site, you may, at some point, need to hook the output of some custom code to appear after your post's content. Thankfully, you can easily…
Read More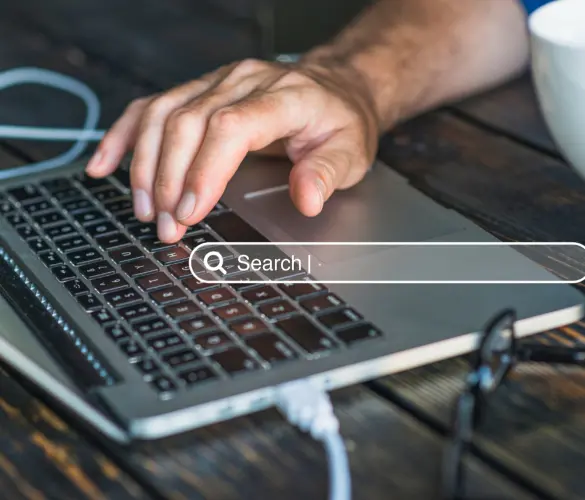
How to... / 5 min read
How to... / 5 min read
How to Search for Slugs in a WordPress Website (5 Methods)
Whether you’re optimizing your website for SEO, troubleshooting errors, or reorganizing content, knowing how to search for slugs in a WordPress website can save you a lot of time and…
Read More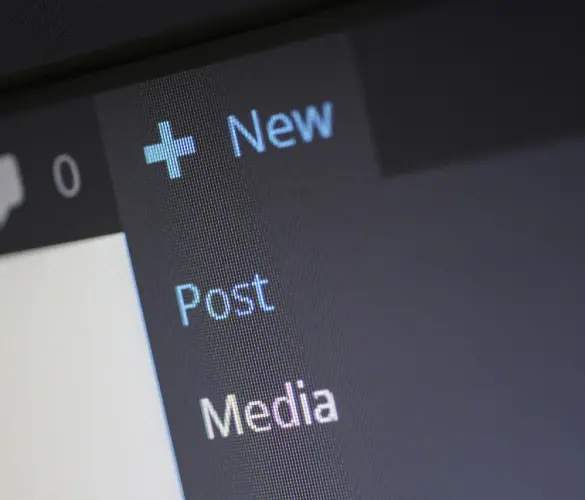
How to... / 11 min read
How to... / 11 min read
Can You Run Multiple Blogs on WordPress?
Yes, it is possible for you to run multiple blogs on the same WordPress site at the same time. However, WordPress doesn't support multiple individual blogs by default, so you…
Read More