- Blogs
- Development
- WordPress wp_get_attachment_image: What it Is and How to Use it
Development / 6 min read
WordPress wp_get_attachment_image: What it Is and How to Use it
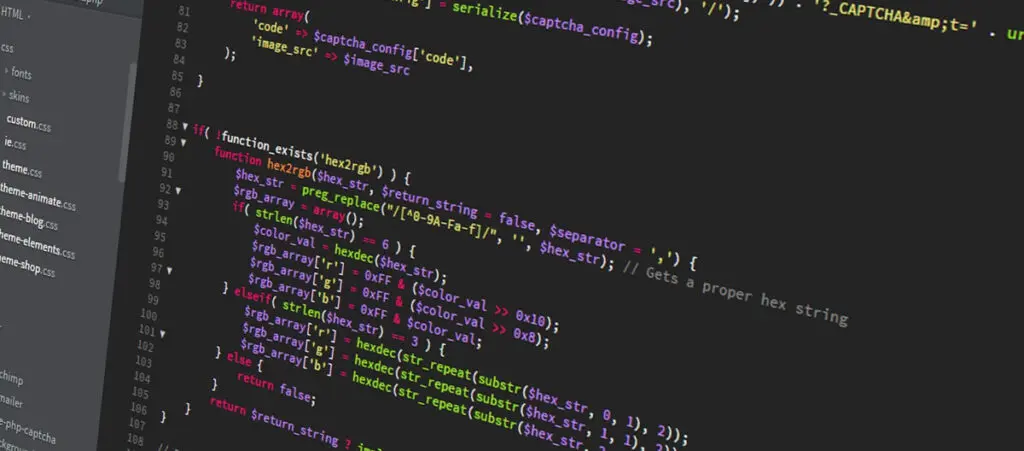
The wp_get_attachment_image helps you retrieve and display HTML img
elements from any image on your WordPress site based on its attachment ID
. Keep reading to understand how it works and how you can use it to dynamically display images on your website.
Key Takeaways
- wp_get_attachment_image takes in an attachment ID and returns a customizable HTML img element to display the image.
- You can use wp_get_attachment_image to display images from anywhere on your site, giving you a lot of flexibility in how you present your visual content.
What is wp_get_attachment_image in WordPress?
wp_get_attachment_image is a WordPress function used to retrieve an HTML image element (img
tag) for a given attachment or media file. Based on the attachment ID
provided, it generates the necessary HTML to display the image.
Developers use wp_get_attachment_image
to retrieve and display image attachments in their WordPress sites.
How Does wp_get_attachment_image Work in WordPress?
wp_get_attachment_image
returns a string with an HTML img
tag for a specified image attachment. But how does it do it? To understand how it works, let’s explore the function’s definition:
wp_get_attachment_image(
int $attachment_id,
string|int[] $size = ‘thumbnail’,
bool $icon = false,
string|array $attr = ”
): string
Let’s break down each parameter:
$attachment_id
$attachment_id
is the only required parameter. It’s an integer that specifies the ID
of the attachment (or media file) for which you want to retrieve the image.
$size
$size
is an optional parameter that determines the size of the image to retrieve. You can specify the size of the retrieved image as a string or an array:
- String. Use a registered image size name like ‘thumbnail’, ‘medium’, and ‘large’ or a custom size name defined via add_image_size().
- Array. You can also pass an array with the format [width, height] to specify a custom size.
The $size
parameter has a default value of ‘thumbnail’
, so the image will display in a thumbnail size unless you specify otherwise.
$icon
$icon
is an optional Boolean parameter that determines how to handle icons. If set to true
, it retrieves the attachment as an icon-sized image. It’s false
by default, which means it retrieves the image at the specified or default ‘thumbnail’
size.
$attr
$attr
is an optional array parameter that allows you to define attributes for the img
tag generated by the function. This parameter takes an associative array where keys represent attribute names (like 'class'
, 'alt'
, 'title'
, etc.) and values represent the attribute’s values.
- src. String parameter that specifies the image attachment URL.
- class. String that specifies the CSS class name or space-separated list of classes.
- alt string. String that specifies the image’s alt attribute.
- srcset. String to specify the URL of the image to use in different situations.
- sizes. String to specify the value of the sizes attribute, which determines the sizes of icons.
- loading. String to determine whether a browser should load an image immediately or only when the user’s viewport gets near the image. A value of ‘lazy’ enables lazy loading, while false results in the attribute being omitted for the image. It defaults to ‘lazy’ and is dependent on wp_lazy_loading_enabled().
- decoding. String to specify the ‘decoding’ attribute value. Possible values are ‘async’ (default), ‘sync’, or ‘auto’. Passing false or an empty string will result in the attribute being omitted.
How to Use wp_get_attachment_image in WordPress?
Now that we’ve explored all the function’s attributes, let’s provide some examples of how to use it on your WordPress site.
Use wp_get_attachment_image to Display Your Post’s Thumbnail
Let’s use wp_get_attachment_image
to retrieve and display the current post’s thumbnail image at the bottom of the post.
Place the following code snippet in your theme’s functions.php
file or create a custom plugin for this feature:
// Function to display the thumbnail picture at the bottom of single posts
function display_thumbnail_image($content){
// Function works only for posts, not pages or any other content type
if ( is_single() && is_main_query() ) {
// Checks whether the post has a thumbnail picture
if ( has_post_thumbnail() ) {
// Get the thumbnail ID and passes as an argument to wp_get_attachment_image
$thumbnail_id = get_post_thumbnail_id();
$image = wp_get_attachment_image( $thumbnail_id, 'medium' );
// Content that will appear at the bottom of the post
$extra_content = '<p><b>The ID of this post\'s thumbnail is: ' . $thumbnail_id . '</b></p>' . '<p>' . $image . '</p>';
// Append the new content to the post's content
$content .= $extra_content;
}
}
return $content;
}
add_filter( 'the_content', 'display_thumbnail_image' );
Here’s how this function works:
- First, it checks whether the current content being displayed is a post.
- If it is, it checks whether the post has a featured image.
- If it does, it retrieves the image’s ID with get_post_thumbnail_id.
- It passes the retrieved ID into wp_get_attachment_image.
- It appends some text and the HTML img element to the bottom of the post.
This is the post before applying this function:
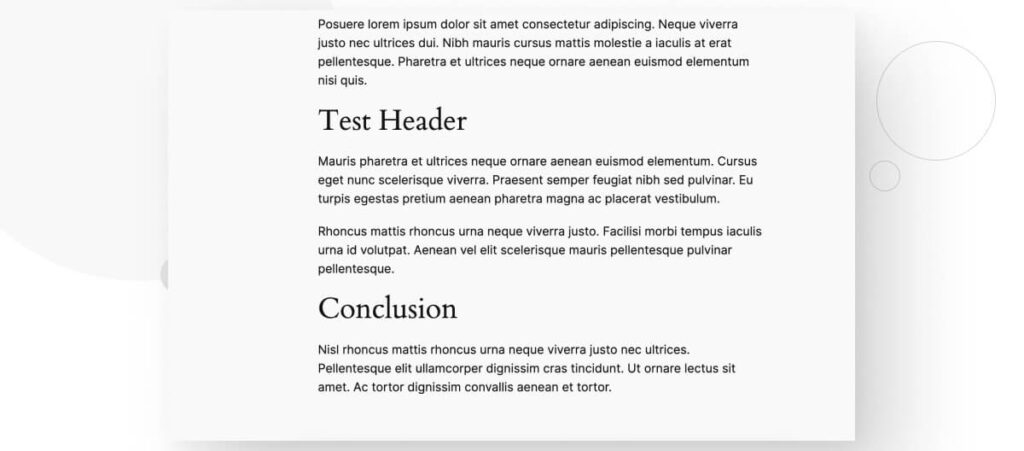
And this is what it looks like after applying the function:
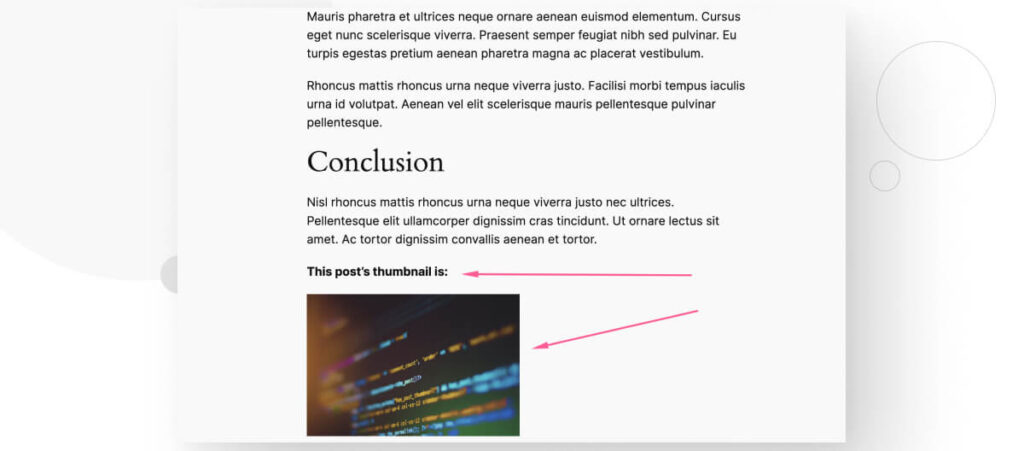
Display All Images From A Specific Post With A Custom Size
Similar to the previous function, you can display all images from a specific post at the bottom of every post on your site using wp_get_attachment_image
. To do it, use the following code snippet:
// Function to retrieve and display all images from a specific post at the bottom of single posts
function display_all_images_from_post($content) {
// Function works only for posts, not pages or any other content type
if ( is_single() && is_main_query() ) {
// Specify a post ID and get all images uploaded to that post
$post_ID = 10;
$post_images = get_attached_media( 'image', $post_ID );
// Create the text that will appear at the bottom of every post
$extra_content = '<p><b>These are all the images from post ID ' . $post_ID . ' :</b></p>';
if ( !empty( $post_images )) {
foreach ( $post_images as $image ) {
// Append each image as a separate paragraph, one after the other
$extra_content .= '<p>' . wp_get_attachment_image( $image->ID, [250, 250] ) . '<p>';
}
// Append the text and the images at the bottom of the post
$content .= $extra_content;
return $content;
}
}
}
add_filter( 'the_content', 'display_all_images_from_post' );
Here’s what it looks like on your live site:
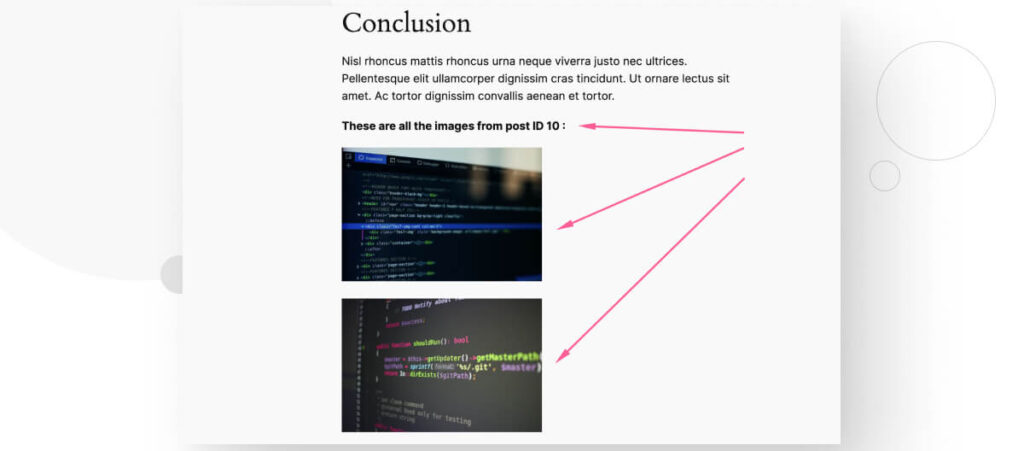
wp_get_attachment_image Helps You Retrieve and Display Images From All Over Your Site
wp_get_attachment_image
is a WordPress function that allows you to retrieve and display an HTML img
element from every image on your site.
Using this function in combination with functions that allow you to retrieve data like post IDs and image attachments from other posts gives you a lot of flexibility when displaying images on your site.
Hopefully, this post helped you understand the wp_get_attachment_image
function and gave you some ideas on how to use it on your site.
If you found this post useful, read our blog and resources for more insights and guides!
Related Articles
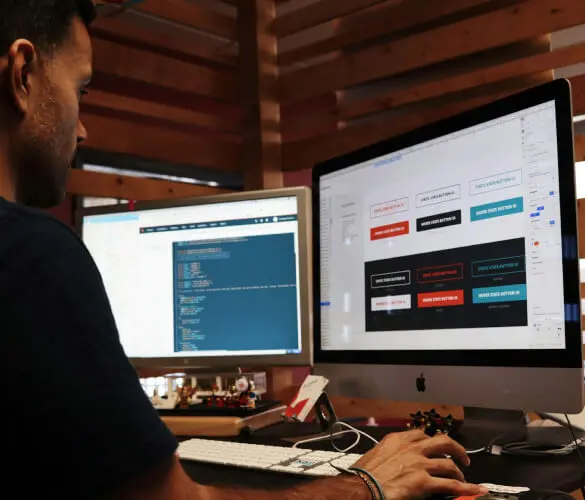
Development / 10 min read
Development / 10 min read
How to Change or Edit Your WordPress Footer
If you’re starting to dive deeper into your WordPress site, you may be wondering how to change or edit the contents of your footer. After all, it’s the part of…
Read More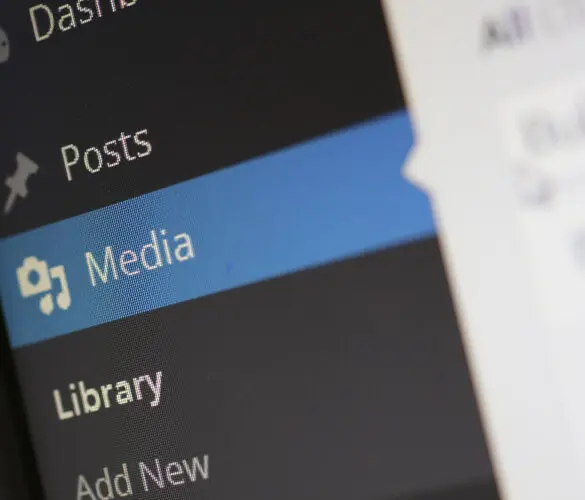
Development / 8 min read
Development / 8 min read
How to Change the Size of Your Featured Image in WordPress
The size of the featured image in WordPress is an important factor in the look and feel of your posts, pages, and custom post types. As a result, you may…
Read More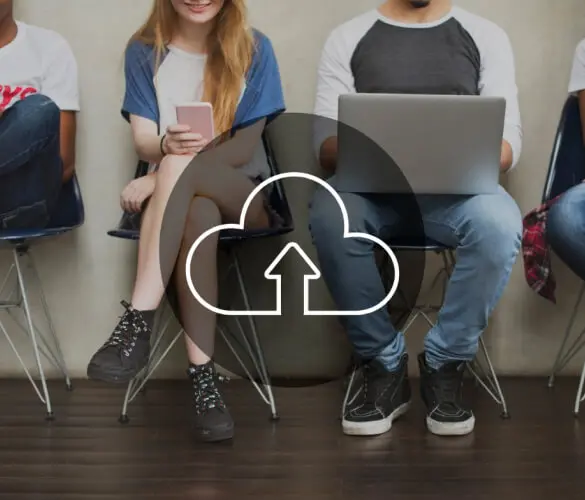
Development / 7 min read
Development / 7 min read
How to Clean Up your WordPress Uploads Folder?
The WordPress Uploads folder is located on wp-content and contains all media files, such as images, videos, and documents, that you upload through the WordPress Media Library. Over time, it…
Read More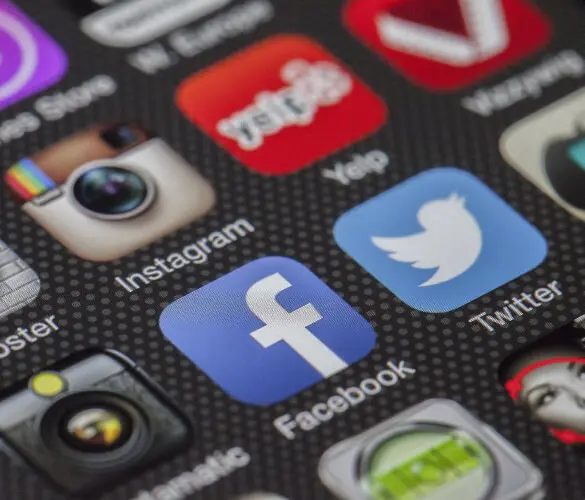
Development / 2 min read
Development / 2 min read
How to Change the Color of Social Media Icons in WordPress?
Looking for how to change the color of social media icons in WordPress? By default, social media icons in WordPress have unique colors. This can be great, but it can…
Read More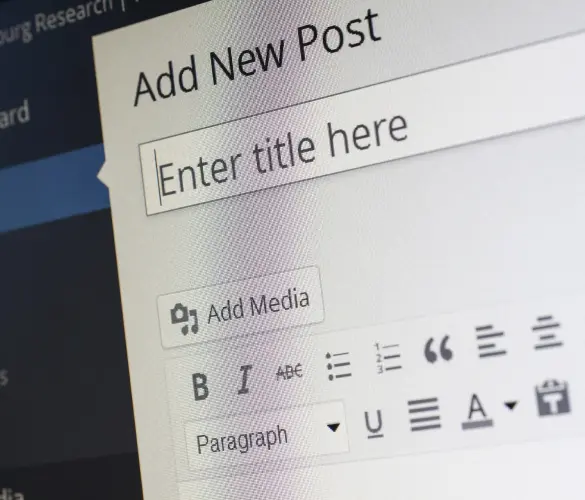
Development / 7 min read
Development / 7 min read
How to Hide the Page and Post Title in WordPress
Do you want to hide your post or page title in WordPress? Sometimes, it may be necessary to do it, especially if you want your home page or informational pages…
Read More